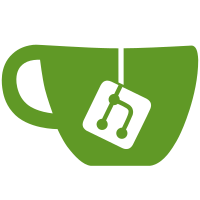
git-svn-id: https://free-cad.svn.sourceforge.net/svnroot/free-cad/trunk@5000 e8eeb9e2-ec13-0410-a4a9-efa5cf37419d
92 lines
3.7 KiB
C++
92 lines
3.7 KiB
C++
/***************************************************************************
|
|
* Copyright (c) 2010 Juergen Riegel <FreeCAD@juergen-riegel.net> *
|
|
* *
|
|
* This file is part of the FreeCAD CAx development system. *
|
|
* *
|
|
* This library is free software; you can redistribute it and/or *
|
|
* modify it under the terms of the GNU Library General Public *
|
|
* License as published by the Free Software Foundation; either *
|
|
* version 2 of the License, or (at your option) any later version. *
|
|
* *
|
|
* This library is distributed in the hope that it will be useful, *
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of *
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the *
|
|
* GNU Library General Public License for more details. *
|
|
* *
|
|
* You should have received a copy of the GNU Library General Public *
|
|
* License along with this library; see the file COPYING.LIB. If not, *
|
|
* write to the Free Software Foundation, Inc., 59 Temple Place, *
|
|
* Suite 330, Boston, MA 02111-1307, USA *
|
|
* *
|
|
***************************************************************************/
|
|
|
|
|
|
#include "PreCompiled.h"
|
|
#ifndef _PreComp_
|
|
# include <TopExp_Explorer.hxx>
|
|
# include <TopoDS.hxx>
|
|
# include <TopoDS_Edge.hxx>
|
|
#endif
|
|
|
|
#include <Mod/Part/App/TopoShape.h>
|
|
|
|
#include "FeatureChamfer.h"
|
|
|
|
|
|
using namespace PartDesign;
|
|
|
|
|
|
PROPERTY_SOURCE(PartDesign::Chamfer, PartDesign::DressUp)
|
|
|
|
Chamfer::Chamfer()
|
|
{
|
|
ADD_PROPERTY(Size,(1.0f));
|
|
}
|
|
|
|
short Chamfer::mustExecute() const
|
|
{
|
|
if (Base.isTouched() || Size.isTouched())
|
|
return 1;
|
|
if (Base.getValue() && Base.getValue()->isTouched())
|
|
return 1;
|
|
return 0;
|
|
}
|
|
|
|
App::DocumentObjectExecReturn *Chamfer::execute(void)
|
|
{
|
|
/* App::DocumentObject* link = Base.getValue();
|
|
if (!link)
|
|
return new App::DocumentObjectExecReturn("No object linked");
|
|
if (!link->getTypeId().isDerivedFrom(Part::Feature::getClassTypeId()))
|
|
return new App::DocumentObjectExecReturn("Linked object is not a Part object");
|
|
Part::Feature *base = static_cast<Part::Feature*>(Base.getValue());
|
|
const Part::TopoShape& TopShape = base->Shape.getShape();
|
|
|
|
const std::vector<std::string>& SubVals = Base.getSubValuesStartsWith("Edge");
|
|
if (SubVals.size() == 0)
|
|
return new App::DocumentObjectExecReturn("No Edges specified");
|
|
|
|
float radius = Radius.getValue();
|
|
|
|
try {
|
|
BRepChamferAPI_MakeChamfer mkChamfer(base->Shape.getValue());
|
|
|
|
for (std::vector<std::string>::const_iterator it= SubVals.begin();it!=SubVals.end();++it) {
|
|
TopoDS_Edge edge = TopoDS::Edge(TopShape.getSubShape(it->c_str()));
|
|
mkChamfer.Add(radius, radius, edge);
|
|
}
|
|
|
|
TopoDS_Shape shape = mkChamfer.Shape();
|
|
if (shape.IsNull())
|
|
return new App::DocumentObjectExecReturn("Resulting shape is null");
|
|
this->Shape.setValue(shape);
|
|
return App::DocumentObject::StdReturn;
|
|
}
|
|
catch (Standard_Failure) {
|
|
Handle_Standard_Failure e = Standard_Failure::Caught();
|
|
return new App::DocumentObjectExecReturn(e->GetMessageString());
|
|
}*/
|
|
|
|
return App::DocumentObject::StdReturn;
|
|
}
|