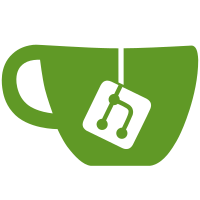
I didn't know BRepAdaptor_Curve does not take shape orientation (reverseness) into account. The commit can break existing projects. If revolution feature was created with axis linked to reversed edge, and angle span is not 360, the revolution direction will now swap. The chances of this situation are pretty low, and revolution supports axis linkage for not long yet. So I hope it won't cause any noticeable trouble. --DeepSOIC
180 lines
7.3 KiB
C++
180 lines
7.3 KiB
C++
/***************************************************************************
|
|
* Copyright (c) 2009 Werner Mayer <wmayer[at]users.sourceforge.net> *
|
|
* *
|
|
* This file is part of the FreeCAD CAx development system. *
|
|
* *
|
|
* This library is free software; you can redistribute it and/or *
|
|
* modify it under the terms of the GNU Library General Public *
|
|
* License as published by the Free Software Foundation; either *
|
|
* version 2 of the License, or (at your option) any later version. *
|
|
* *
|
|
* This library is distributed in the hope that it will be useful, *
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of *
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the *
|
|
* GNU Library General Public License for more details. *
|
|
* *
|
|
* You should have received a copy of the GNU Library General Public *
|
|
* License along with this library; see the file COPYING.LIB. If not, *
|
|
* write to the Free Software Foundation, Inc., 59 Temple Place, *
|
|
* Suite 330, Boston, MA 02111-1307, USA *
|
|
* *
|
|
***************************************************************************/
|
|
|
|
|
|
#include "PreCompiled.h"
|
|
#ifndef _PreComp_
|
|
# include <gp_Ax1.hxx>
|
|
# include <TopoDS.hxx>
|
|
# include <BRepAdaptor_Curve.hxx>
|
|
# include <gp_Lin.hxx>
|
|
# include <gp_Circ.hxx>
|
|
#endif
|
|
|
|
|
|
#include "FeatureRevolution.h"
|
|
#include <Base/Tools.h>
|
|
#include <Base/Exception.h>
|
|
#include <App/Application.h>
|
|
|
|
using namespace Part;
|
|
|
|
App::PropertyFloatConstraint::Constraints Revolution::angleRangeU = {-360.0,360.0,1.0};
|
|
|
|
PROPERTY_SOURCE(Part::Revolution, Part::Feature)
|
|
|
|
Revolution::Revolution()
|
|
{
|
|
ADD_PROPERTY_TYPE(Source,(0), "Revolve", App::Prop_None, "Shape to revolve");
|
|
ADD_PROPERTY_TYPE(Base,(Base::Vector3d(0.0,0.0,0.0)), "Revolve", App::Prop_None, "Base point of revolution axis");
|
|
ADD_PROPERTY_TYPE(Axis,(Base::Vector3d(0.0,0.0,1.0)), "Revolve", App::Prop_None, "Direction of revolution axis");
|
|
ADD_PROPERTY_TYPE(AxisLink,(0),"Revolve",App::Prop_None,"Link to edge to use as revolution axis.");
|
|
ADD_PROPERTY_TYPE(Angle,(360.0), "Revolve", App::Prop_None, "Angle span of revolution. If angle is zero, and an arc is used for axis link, angle span of arc will be used.");
|
|
Angle.setConstraints(&angleRangeU);
|
|
ADD_PROPERTY_TYPE(Symmetric,(false),"Revolve",App::Prop_None,"Extend revolution symmetrically from the profile.");
|
|
ADD_PROPERTY_TYPE(Solid,(false),"Revolve",App::Prop_None,"Make revolution a solid if possible");
|
|
}
|
|
|
|
short Revolution::mustExecute() const
|
|
{
|
|
if (Base.isTouched() ||
|
|
Axis.isTouched() ||
|
|
Angle.isTouched() ||
|
|
Source.isTouched() ||
|
|
Solid.isTouched() ||
|
|
AxisLink.isTouched() ||
|
|
Symmetric.isTouched())
|
|
return 1;
|
|
return 0;
|
|
}
|
|
|
|
void Revolution::onChanged(const App::Property* prop)
|
|
{
|
|
if(! this->isRestoring()){
|
|
if(prop == &AxisLink){
|
|
Base.setReadOnly(AxisLink.getValue() != nullptr);
|
|
Axis.setReadOnly(AxisLink.getValue() != nullptr);
|
|
}
|
|
}
|
|
Part::Feature::onChanged(prop);
|
|
}
|
|
|
|
bool Revolution::fetchAxisLink(const App::PropertyLinkSub &axisLink,
|
|
Base::Vector3d& center,
|
|
Base::Vector3d& dir,
|
|
double& angle)
|
|
{
|
|
if (!axisLink.getValue())
|
|
return false;
|
|
|
|
if (!axisLink.getValue()->isDerivedFrom(Part::Feature::getClassTypeId()))
|
|
throw Base::TypeError("AxisLink has no OCC shape");
|
|
|
|
Part::Feature* linked = static_cast<Part::Feature*>(axisLink.getValue());
|
|
|
|
TopoDS_Shape axEdge;
|
|
if (axisLink.getSubValues().size() > 0 && axisLink.getSubValues()[0].length() > 0){
|
|
axEdge = linked->Shape.getShape().getSubShape(axisLink.getSubValues()[0].c_str());
|
|
} else {
|
|
axEdge = linked->Shape.getValue();
|
|
}
|
|
|
|
if (axEdge.IsNull())
|
|
throw Base::ValueError("AxisLink shape is null");
|
|
if (axEdge.ShapeType() != TopAbs_EDGE)
|
|
throw Base::TypeError("AxisLink shape is not an edge");
|
|
|
|
BRepAdaptor_Curve crv(TopoDS::Edge(axEdge));
|
|
gp_Pnt base;
|
|
gp_Dir occdir;
|
|
bool reversed = axEdge.Orientation() == TopAbs_REVERSED;
|
|
if (crv.GetType() == GeomAbs_Line){
|
|
base = crv.Value(reversed ? crv.FirstParameter() : crv.LastParameter());
|
|
occdir = crv.Line().Direction();
|
|
} else if (crv.GetType() == GeomAbs_Circle) {
|
|
base = crv.Circle().Axis().Location();
|
|
occdir = crv.Circle().Axis().Direction();
|
|
angle = crv.LastParameter() - crv.FirstParameter();
|
|
} else {
|
|
throw Base::TypeError("AxisLink edge is neither line nor arc of circle.");
|
|
}
|
|
if (reversed)
|
|
occdir.Reverse();
|
|
center.Set(base.X(), base.Y(),base.Z());
|
|
dir.Set(occdir.X(), occdir.Y(), occdir.Z());
|
|
return true;
|
|
}
|
|
|
|
App::DocumentObjectExecReturn *Revolution::execute(void)
|
|
{
|
|
App::DocumentObject* link = Source.getValue();
|
|
if (!link)
|
|
return new App::DocumentObjectExecReturn("No object linked");
|
|
if (!link->getTypeId().isDerivedFrom(Part::Feature::getClassTypeId()))
|
|
return new App::DocumentObjectExecReturn("Linked object is not a Part object");
|
|
Part::Feature *base = static_cast<Part::Feature*>(Source.getValue());
|
|
|
|
try {
|
|
//read out axis link
|
|
double angle_edge = 0;
|
|
Base::Vector3d b = Base.getValue();
|
|
Base::Vector3d v = Axis.getValue();
|
|
bool linkFetched = this->fetchAxisLink(this->AxisLink, b, v, angle_edge);
|
|
if (linkFetched){
|
|
this->Base.setValue(b);
|
|
this->Axis.setValue(v);
|
|
}
|
|
|
|
gp_Pnt pnt(b.x,b.y,b.z);
|
|
gp_Dir dir(v.x,v.y,v.z);
|
|
gp_Ax1 revAx(pnt, dir);
|
|
|
|
//read out revolution angle
|
|
double angle = Angle.getValue()/180.0f*M_PI;
|
|
if (fabs(angle) < Precision::Angular())
|
|
angle = angle_edge;
|
|
|
|
//apply "midplane" symmetry
|
|
TopoShape sourceShape = base->Shape.getShape();
|
|
if (Symmetric.getValue()) {
|
|
//rotate source shape backwards by half angle, to make resulting revolution symmetric to the profile
|
|
gp_Trsf mov;
|
|
mov.SetRotation(revAx, angle * (-0.5));
|
|
TopLoc_Location loc(mov);
|
|
sourceShape.setShape(sourceShape.getShape().Moved(loc));
|
|
}
|
|
|
|
//do it!
|
|
Standard_Boolean makeSolid = Solid.getValue() ? Standard_True : Standard_False;
|
|
TopoDS_Shape revolve = sourceShape.revolve(revAx, angle, makeSolid);
|
|
|
|
if (revolve.IsNull())
|
|
return new App::DocumentObjectExecReturn("Resulting shape is null");
|
|
this->Shape.setValue(revolve);
|
|
return App::DocumentObject::StdReturn;
|
|
}
|
|
catch (Standard_Failure) {
|
|
Handle_Standard_Failure e = Standard_Failure::Caught();
|
|
return new App::DocumentObjectExecReturn(e->GetMessageString());
|
|
}
|
|
}
|