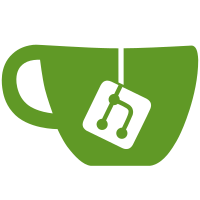
git-svn-id: https://free-cad.svn.sourceforge.net/svnroot/free-cad/trunk@5000 e8eeb9e2-ec13-0410-a4a9-efa5cf37419d
96 lines
6.2 KiB
Python
96 lines
6.2 KiB
Python
# -*- coding: utf-8 -*-
|
|
|
|
# Form implementation generated from reading ui file 'postprocess.ui'
|
|
#
|
|
# Created: Wed Jan 26 13:34:56 2011
|
|
# by: PyQt4 UI code generator 4.7.4
|
|
#
|
|
# WARNING! All changes made in this file will be lost!
|
|
|
|
from PyQt4 import QtCore, QtGui
|
|
|
|
class Ui_dialog(object):
|
|
def setupUi(self, dialog):
|
|
dialog.setObjectName("dialog")
|
|
dialog.resize(380, 170)
|
|
sizePolicy = QtGui.QSizePolicy(QtGui.QSizePolicy.Expanding, QtGui.QSizePolicy.Expanding)
|
|
sizePolicy.setHorizontalStretch(0)
|
|
sizePolicy.setVerticalStretch(0)
|
|
sizePolicy.setHeightForWidth(dialog.sizePolicy().hasHeightForWidth())
|
|
dialog.setSizePolicy(sizePolicy)
|
|
dialog.setMinimumSize(QtCore.QSize(0, 0))
|
|
self.gridLayout_2 = QtGui.QGridLayout(dialog)
|
|
self.gridLayout_2.setObjectName("gridLayout_2")
|
|
self.groupBox_2 = QtGui.QGroupBox(dialog)
|
|
self.groupBox_2.setObjectName("groupBox_2")
|
|
self.gridLayout = QtGui.QGridLayout(self.groupBox_2)
|
|
self.gridLayout.setObjectName("gridLayout")
|
|
self.check_fly_to_buy_7 = QtGui.QCheckBox(self.groupBox_2)
|
|
self.check_fly_to_buy_7.setMinimumSize(QtCore.QSize(148, 18))
|
|
self.check_fly_to_buy_7.setChecked(False)
|
|
self.check_fly_to_buy_7.setObjectName("check_fly_to_buy_7")
|
|
self.gridLayout.addWidget(self.check_fly_to_buy_7, 0, 0, 1, 1)
|
|
self.check_fly_to_buy_4 = QtGui.QCheckBox(self.groupBox_2)
|
|
self.check_fly_to_buy_4.setMinimumSize(QtCore.QSize(148, 18))
|
|
self.check_fly_to_buy_4.setChecked(False)
|
|
self.check_fly_to_buy_4.setObjectName("check_fly_to_buy_4")
|
|
self.gridLayout.addWidget(self.check_fly_to_buy_4, 0, 1, 1, 1)
|
|
spacerItem = QtGui.QSpacerItem(20, 40, QtGui.QSizePolicy.Minimum, QtGui.QSizePolicy.Expanding)
|
|
self.gridLayout.addItem(spacerItem, 1, 0, 1, 1)
|
|
spacerItem1 = QtGui.QSpacerItem(20, 40, QtGui.QSizePolicy.Minimum, QtGui.QSizePolicy.Expanding)
|
|
self.gridLayout.addItem(spacerItem1, 1, 1, 1, 1)
|
|
self.check_fly_to_buy_8 = QtGui.QCheckBox(self.groupBox_2)
|
|
self.check_fly_to_buy_8.setMinimumSize(QtCore.QSize(148, 18))
|
|
self.check_fly_to_buy_8.setChecked(False)
|
|
self.check_fly_to_buy_8.setObjectName("check_fly_to_buy_8")
|
|
self.gridLayout.addWidget(self.check_fly_to_buy_8, 2, 0, 1, 1)
|
|
self.check_fly_to_buy_2 = QtGui.QCheckBox(self.groupBox_2)
|
|
self.check_fly_to_buy_2.setMinimumSize(QtCore.QSize(148, 18))
|
|
self.check_fly_to_buy_2.setChecked(False)
|
|
self.check_fly_to_buy_2.setObjectName("check_fly_to_buy_2")
|
|
self.gridLayout.addWidget(self.check_fly_to_buy_2, 2, 1, 1, 1)
|
|
spacerItem2 = QtGui.QSpacerItem(20, 40, QtGui.QSizePolicy.Minimum, QtGui.QSizePolicy.Expanding)
|
|
self.gridLayout.addItem(spacerItem2, 3, 0, 1, 1)
|
|
spacerItem3 = QtGui.QSpacerItem(20, 40, QtGui.QSizePolicy.Minimum, QtGui.QSizePolicy.Expanding)
|
|
self.gridLayout.addItem(spacerItem3, 3, 1, 1, 1)
|
|
self.check_fly_to_buy_5 = QtGui.QCheckBox(self.groupBox_2)
|
|
self.check_fly_to_buy_5.setMinimumSize(QtCore.QSize(148, 18))
|
|
self.check_fly_to_buy_5.setChecked(False)
|
|
self.check_fly_to_buy_5.setObjectName("check_fly_to_buy_5")
|
|
self.gridLayout.addWidget(self.check_fly_to_buy_5, 4, 0, 1, 1)
|
|
self.check_fly_to_buy_3 = QtGui.QCheckBox(self.groupBox_2)
|
|
self.check_fly_to_buy_3.setMinimumSize(QtCore.QSize(148, 18))
|
|
self.check_fly_to_buy_3.setChecked(False)
|
|
self.check_fly_to_buy_3.setObjectName("check_fly_to_buy_3")
|
|
self.gridLayout.addWidget(self.check_fly_to_buy_3, 4, 1, 1, 1)
|
|
self.gridLayout_2.addWidget(self.groupBox_2, 0, 0, 1, 2)
|
|
self.buttonBox = QtGui.QDialogButtonBox(dialog)
|
|
self.buttonBox.setOrientation(QtCore.Qt.Vertical)
|
|
self.buttonBox.setStandardButtons(QtGui.QDialogButtonBox.Cancel|QtGui.QDialogButtonBox.Ok)
|
|
self.buttonBox.setObjectName("buttonBox")
|
|
self.gridLayout_2.addWidget(self.buttonBox, 0, 2, 1, 1)
|
|
self.button_select_results_folder = QtGui.QPushButton(dialog)
|
|
self.button_select_results_folder.setObjectName("button_select_results_folder")
|
|
self.gridLayout_2.addWidget(self.button_select_results_folder, 1, 0, 1, 1)
|
|
self.button_start_postprocessing = QtGui.QPushButton(dialog)
|
|
self.button_start_postprocessing.setEnabled(False)
|
|
self.button_start_postprocessing.setMinimumSize(QtCore.QSize(0, 23))
|
|
self.button_start_postprocessing.setObjectName("button_start_postprocessing")
|
|
self.gridLayout_2.addWidget(self.button_start_postprocessing, 1, 1, 1, 1)
|
|
|
|
self.retranslateUi(dialog)
|
|
QtCore.QMetaObject.connectSlotsByName(dialog)
|
|
|
|
def retranslateUi(self, dialog):
|
|
dialog.setWindowTitle(QtGui.QApplication.translate("dialog", "Machining Distortion Prediction", None, QtGui.QApplication.UnicodeUTF8))
|
|
self.groupBox_2.setTitle(QtGui.QApplication.translate("dialog", "Output Elements", None, QtGui.QApplication.UnicodeUTF8))
|
|
self.check_fly_to_buy_7.setText(QtGui.QApplication.translate("dialog", "Max Displacement X", None, QtGui.QApplication.UnicodeUTF8))
|
|
self.check_fly_to_buy_4.setText(QtGui.QApplication.translate("dialog", "Min Displacement X", None, QtGui.QApplication.UnicodeUTF8))
|
|
self.check_fly_to_buy_8.setText(QtGui.QApplication.translate("dialog", "Max Displacement Y", None, QtGui.QApplication.UnicodeUTF8))
|
|
self.check_fly_to_buy_2.setText(QtGui.QApplication.translate("dialog", "Min Displacement Y", None, QtGui.QApplication.UnicodeUTF8))
|
|
self.check_fly_to_buy_5.setText(QtGui.QApplication.translate("dialog", "Max Displacement Z", None, QtGui.QApplication.UnicodeUTF8))
|
|
self.check_fly_to_buy_3.setText(QtGui.QApplication.translate("dialog", "Min Displacement Z", None, QtGui.QApplication.UnicodeUTF8))
|
|
self.button_select_results_folder.setText(QtGui.QApplication.translate("dialog", "Select Results Folder", None, QtGui.QApplication.UnicodeUTF8))
|
|
self.button_start_postprocessing.setText(QtGui.QApplication.translate("dialog", "Start Postprocessing", None, QtGui.QApplication.UnicodeUTF8))
|
|
|