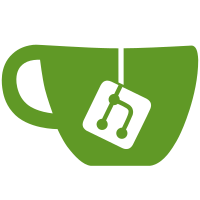
Summary: This diff does a couple different things: - There is now a metrics/ folder, which contains the property files describing the metrics if the fonts, as well as a script for reading and printing the metrics in javascript. - Fractions and superscripts/subscripts are now rendered in slightly different ways now (notably, no use of inline-table). This allows for much more precise positioning of the superscripts, subscripts, numerators, and denominators, while still having an appropriate baseline. Also, there is no longer a sup/sub/supsub distinction, there are only supsubs with null sup/sub. - Using the new font metrics and by implementing the formulas found in The TeX Book, Appendix G, the heights and depths of all of the sub-expressions in a formula are now calculated. These are currently used to: - Correctly position superscripts, subscripts, numerators, and denominators - Adjust the height and depth of the overall expression so it takes up the appropriate space - Because we have to add attributes (height and depth) to every attribute, I have changed the way DOM nodes are assembled. Now, instead of assembling the DOM elements inline (which is a problem because we need to track height/depth, and we shouldn't (and can't in IE 8) attach raw attributes to DOM nodes), we assemble a pseudo-DOM structure with the extra information, and then actually assemble it at the very end. The main page also now has an updated expression to show off and test the new and improved parsing. Test Plan: View the main page, make sure that the expression renders. Make sure that the tests pass. Make sure that expressions have the correct calculated height (this is most easily tested by viewing them on the main page and making sure that the top of the expression lines up with the bottom of the input box). Reviewers: alpert Reviewed By: alpert Differential Revision: http://phabricator.khanacademy.org/D3442
52 lines
1.3 KiB
JavaScript
52 lines
1.3 KiB
JavaScript
var path = require("path");
|
|
|
|
var browserify = require("browserify");
|
|
var express = require("express");
|
|
|
|
var app = express();
|
|
|
|
app.use(express.logger());
|
|
|
|
app.get("/katex.js", function(req, res, next) {
|
|
var b = browserify();
|
|
b.add("./katex");
|
|
|
|
var stream = b.bundle({standalone: "katex"});
|
|
|
|
var body = "";
|
|
stream.on("data", function(s) { body += s; });
|
|
stream.on("error", function(e) { next(e); });
|
|
stream.on("end", function() {
|
|
res.setHeader("Content-Type", "text/javascript");
|
|
res.send(body);
|
|
});
|
|
});
|
|
|
|
app.get("/test/katex-tests.js", function(req, res, next) {
|
|
var b = browserify();
|
|
b.add("./test/katex-tests");
|
|
|
|
var stream = b.bundle({});
|
|
|
|
var body = "";
|
|
stream.on("data", function(s) { body += s; });
|
|
stream.on("error", function(e) { next(e); });
|
|
stream.on("end", function() {
|
|
res.setHeader("Content-Type", "text/javascript");
|
|
res.send(body);
|
|
});
|
|
});
|
|
|
|
app.use(express.static(path.join(__dirname, "static")));
|
|
app.use(express.static(path.join(__dirname, "build")));
|
|
app.use("/test", express.static(path.join(__dirname, "test")));
|
|
|
|
app.use(function(err, req, res, next) {
|
|
console.error(err.stack);
|
|
res.setHeader("Content-Type", "text/plain");
|
|
res.send(500, err.stack);
|
|
});
|
|
|
|
app.listen(7936);
|
|
console.log("Serving on http://0.0.0.0:7936/ ...");
|