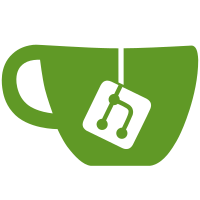
Summary: Add support for math-mode accents. This involves a couple changes. First, in order to correctly position the accents, we must know the kern between every character and the "skewchar" in that font. To do this, we improve our tfm parser to run the mini-kern-language and calculate kerns. We then export these into fontMetrics.js. Then, we add normal support for accents. In particular, we do some special handling for supsubs around accents. This involves building the supsub separately without the accent, and then replacing its base with the built accent. Finally, the character in the fonts for the \vec command is a combining unicode character, so it is shifted to the left, but none of the other characters do this. We add some special handling for \vec to account for this. Fixes #7 Test Plan: - Make sure tests pass - Make sure no huxley screenshots changed, and the new one looks good Reviewers: alpert Reviewed By: alpert Differential Revision: http://phabricator.khanacademy.org/D13157
54 lines
1.3 KiB
Python
Executable File
54 lines
1.3 KiB
Python
Executable File
#!/usr/bin/env python
|
|
|
|
import fontforge
|
|
import sys
|
|
import json
|
|
|
|
metrics_to_extract = {
|
|
"Main-Regular": [
|
|
u"\u2260", # \neq
|
|
u"\u2245", # \cong
|
|
u"\u0020", # space
|
|
u"\u00a0", # nbsp
|
|
u"\u2026", # \ldots
|
|
u"\u22ef", # \cdots
|
|
u"\u22f1", # \ddots
|
|
u"\u22ee", # \vdots
|
|
]
|
|
}
|
|
|
|
|
|
def main():
|
|
start_json = json.load(sys.stdin)
|
|
|
|
for font, chars in metrics_to_extract.iteritems():
|
|
fontInfo = fontforge.open("../static/fonts/KaTeX_" + font + ".ttf")
|
|
|
|
for glyph in fontInfo.glyphs():
|
|
try:
|
|
char = unichr(glyph.unicode)
|
|
except ValueError:
|
|
continue
|
|
|
|
if char in chars:
|
|
_, depth, _, height = glyph.boundingBox()
|
|
|
|
depth = -depth
|
|
|
|
# TODO(emily): Figure out a real way to calculate this
|
|
italic = 0
|
|
skew = 0
|
|
|
|
start_json[font][ord(char)] = {
|
|
height: height / fontInfo.em,
|
|
depth: depth / fontInfo.em,
|
|
italic: italic / fontInfo.em,
|
|
skew: skew / fontInfo.em,
|
|
}
|
|
|
|
sys.stdout.write(
|
|
json.dumps(start_json, separators=(',', ':'), sort_keys=True))
|
|
|
|
if __name__ == "__main__":
|
|
main()
|