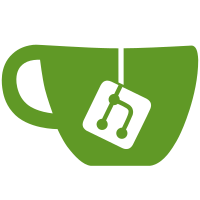
Summary: In LaTeX, large delimiters are the same font size as they are at a normal size, regardless of the actual size. This means that we need to scale up the font size in the inner nodes, which is annoying because we run into the same problem we had with \Huge, etc in those nodes. Thus, this fixes both problems at once. The problem was that when we used our baseline-align-hack and then increased the font size inside of one of the middle (display: block and height: 0) nodes, the node with the increased font size would shift downards (misaligning its baseline). To fix this, we add a method for calculating the maximum font size used in each of the nodes, and adding a small node with this font size to each of the other nodes (including the fix-ie node). This shifts all of the nodes down the same amount, and gets their baselines aligned. Test Plan: - Do dumb things by putting \Huge and \big in places they shouldn't be, and make sure they behave responsibly - Do the same thing in IE 8, 9, 10, 11, Safari, Firefox, and make sure they all behave the same (to some approximation) - Make sure the new huxley image looks good, and the images that changed don't have significant changes Reviewers: alpert Reviewed By: alpert Differential Revision: http://phabricator.khanacademy.org/D12684
49 lines
1.2 KiB
JavaScript
49 lines
1.2 KiB
JavaScript
function Options(style, size, color, parentStyle, parentSize) {
|
|
this.style = style;
|
|
this.color = color;
|
|
this.size = size;
|
|
|
|
if (parentStyle === undefined) {
|
|
parentStyle = style;
|
|
}
|
|
this.parentStyle = parentStyle;
|
|
|
|
if (parentSize === undefined) {
|
|
parentSize = size;
|
|
}
|
|
this.parentSize = parentSize;
|
|
}
|
|
|
|
Options.prototype.withStyle = function(style) {
|
|
return new Options(style, this.size, this.color, this.style, this.size);
|
|
};
|
|
|
|
Options.prototype.withSize = function(size) {
|
|
return new Options(this.style, size, this.color, this.style, this.size);
|
|
};
|
|
|
|
Options.prototype.withColor = function(color) {
|
|
return new Options(this.style, this.size, color, this.style, this.size);
|
|
};
|
|
|
|
Options.prototype.reset = function() {
|
|
return new Options(
|
|
this.style, this.size, this.color, this.style, this.size);
|
|
};
|
|
|
|
var colorMap = {
|
|
"katex-blue": "#6495ed",
|
|
"katex-orange": "#ffa500",
|
|
"katex-pink": "#ff00af",
|
|
"katex-red": "#df0030",
|
|
"katex-green": "#28ae7b",
|
|
"katex-gray": "gray",
|
|
"katex-purple": "#9d38bd"
|
|
};
|
|
|
|
Options.prototype.getColor = function() {
|
|
return colorMap[this.color] || this.color;
|
|
};
|
|
|
|
module.exports = Options;
|