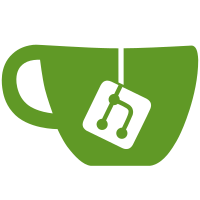
Summary: This adds support for rendering KaTeX to both HTML and MathML with the intent of improving accessibility. To accomplish this, both MathML and HTML are rendered, but with the MathML visually hidden and the HTML spans aria-hidden. Hopefully, this should produce much better accessibility for KaTeX. Should fix/improve #38 Closes #189 Test Plan: - Ensure all the tests, and the new tests, still pass. - Ensure that for each of the group types in `buildHTML.js`, there is a corresponding one in `buildMathML.js`. - Ensure that the huxley screenshots didn't change (except for BinomTest, which changed because I fixed a bug in `buildHTML` where `genfrac` didn't have a `groupToType` mapping). - Run ChromeVox on the test page, render some math. (for example, `\sqrt{x^2}`) - Ensure that a mathy-sounding expression is read. (I hear "group square root of x squared math"). - Ensure that nothing else is read (like no "x" or "2"). - Ensure that MathML markup is generated correctly and is interpreted by the browser correctly by running `document.getElementById("math").innerHTML = katex.renderToString("\\sqrt{x^2}");` and seeing that the same speech is read. Reviewers: john, alpert Reviewed By: john, alpert Subscribers: alpert, john Differential Revision: https://phabricator.khanacademy.org/D16373
60 lines
1.7 KiB
JavaScript
60 lines
1.7 KiB
JavaScript
/**
|
|
* This is the main entry point for KaTeX. Here, we expose functions for
|
|
* rendering expressions either to DOM nodes or to markup strings.
|
|
*
|
|
* We also expose the ParseError class to check if errors thrown from KaTeX are
|
|
* errors in the expression, or errors in javascript handling.
|
|
*/
|
|
|
|
var ParseError = require("./src/ParseError");
|
|
var Settings = require("./src/Settings");
|
|
|
|
var buildTree = require("./src/buildTree");
|
|
var parseTree = require("./src/parseTree");
|
|
var utils = require("./src/utils");
|
|
|
|
/**
|
|
* Parse and build an expression, and place that expression in the DOM node
|
|
* given.
|
|
*/
|
|
var render = function(expression, baseNode, options) {
|
|
utils.clearNode(baseNode);
|
|
|
|
var settings = new Settings(options);
|
|
|
|
var tree = parseTree(expression, settings);
|
|
var node = buildTree(tree, expression, settings).toNode();
|
|
|
|
baseNode.appendChild(node);
|
|
};
|
|
|
|
// KaTeX's styles don't work properly in quirks mode. Print out an error, and
|
|
// disable rendering.
|
|
if (typeof document !== "undefined") {
|
|
if (document.compatMode !== "CSS1Compat") {
|
|
typeof console !== "undefined" && console.warn(
|
|
"Warning: KaTeX doesn't work in quirks mode. Make sure your " +
|
|
"website has a suitable doctype.");
|
|
|
|
render = function() {
|
|
throw new ParseError("KaTeX doesn't work in quirks mode.");
|
|
};
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Parse and build an expression, and return the markup for that.
|
|
*/
|
|
var renderToString = function(expression, options) {
|
|
var settings = new Settings(options);
|
|
|
|
var tree = parseTree(expression, settings);
|
|
return buildTree(tree, expression, settings).toMarkup();
|
|
};
|
|
|
|
module.exports = {
|
|
render: render,
|
|
renderToString: renderToString,
|
|
ParseError: ParseError
|
|
};
|