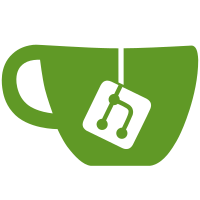
Summary: Create a fonts.less file which generates identical css to fonts.css, but using less rules to be more understandable and customizable. For example, add the ability to change where the fonts directory is located (instead of mandating it be located next to the less file), and add the ability to disable specific font formats (like disable EOTs when IE8 support isn't needed). Test Plan: - Ensure that the test page and huxley page still work - Ensure that the output of `./node_modules/.bin/lessc static/fonts.less` is the same as the original css by running both through `./node_modules/.bin/cleancss` and diffing them. - Ensure that the huxley screenshots haven't changed - Ensure that the build step still works Reviewers: alpert Reviewed By: alpert Differential Revision: http://phabricator.khanacademy.org/D13326
78 lines
1.9 KiB
JavaScript
78 lines
1.9 KiB
JavaScript
var fs = require("fs");
|
|
var path = require("path");
|
|
|
|
var browserify = require("browserify");
|
|
var express = require("express");
|
|
var less = require("less");
|
|
|
|
var app = express();
|
|
|
|
app.use(express.logger());
|
|
|
|
app.get("/katex.js", function(req, res, next) {
|
|
var b = browserify();
|
|
b.add("./katex");
|
|
|
|
var stream = b.bundle({standalone: "katex"});
|
|
|
|
var body = "";
|
|
stream.on("data", function(s) { body += s; });
|
|
stream.on("error", function(e) { next(e); });
|
|
stream.on("end", function() {
|
|
res.setHeader("Content-Type", "text/javascript");
|
|
res.send(body);
|
|
});
|
|
});
|
|
|
|
app.get("/katex.css", function(req, res, next) {
|
|
fs.readFile("static/katex.less", {encoding: "utf8"}, function(err, data) {
|
|
if (err) {
|
|
next(err);
|
|
return;
|
|
}
|
|
|
|
var parser = new less.Parser({
|
|
paths: ["./static"],
|
|
filename: "katex.less"
|
|
});
|
|
|
|
parser.parse(data, function(err, tree) {
|
|
if (err) {
|
|
next(err);
|
|
return;
|
|
}
|
|
|
|
res.setHeader("Content-Type", "text/css");
|
|
res.send(tree.toCSS());
|
|
});
|
|
});
|
|
});
|
|
|
|
app.get("/test/katex-spec.js", function(req, res, next) {
|
|
var b = browserify();
|
|
b.add("./test/katex-spec");
|
|
|
|
var stream = b.bundle({});
|
|
|
|
var body = "";
|
|
stream.on("data", function(s) { body += s; });
|
|
stream.on("error", function(e) { next(e); });
|
|
stream.on("end", function() {
|
|
res.setHeader("Content-Type", "text/javascript");
|
|
res.send(body);
|
|
});
|
|
});
|
|
|
|
app.use(express.static(path.join(__dirname, "static")));
|
|
app.use(express.static(path.join(__dirname, "build")));
|
|
app.use("/test", express.static(path.join(__dirname, "test")));
|
|
|
|
app.use(function(err, req, res, next) {
|
|
console.error(err.stack);
|
|
res.setHeader("Content-Type", "text/plain");
|
|
res.send(500, err.stack);
|
|
});
|
|
|
|
app.listen(7936);
|
|
console.log("Serving on http://0.0.0.0:7936/ ...");
|