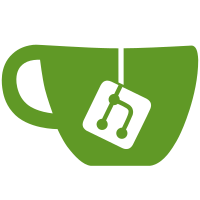
Rename resources to dist. Eliminate keyring bundle and expose keyring class in openpgp module. Add mochaTest grunt task to run node server-side tests. Add node_pack grunt task to create npm package into dist and install it for testing. Add node_store config property which specifies location of localStorage emulation when using node. Add repository info to package.json. Move util.js to src directory from util since it is the only file there. Rename class properties in openpgp to the new class names.
215 lines
13 KiB
HTML
215 lines
13 KiB
HTML
<!DOCTYPE html>
|
|
<html lang="en">
|
|
<head>
|
|
<meta charset="utf-8">
|
|
<title>JSDoc: Source: openpgp.js</title>
|
|
|
|
<script src="scripts/prettify/prettify.js"> </script>
|
|
<script src="scripts/prettify/lang-css.js"> </script>
|
|
<!--[if lt IE 9]>
|
|
<script src="//html5shiv.googlecode.com/svn/trunk/html5.js"></script>
|
|
<![endif]-->
|
|
<link type="text/css" rel="stylesheet" href="styles/prettify-tomorrow.css">
|
|
<link type="text/css" rel="stylesheet" href="styles/jsdoc-default.css">
|
|
</head>
|
|
|
|
<body>
|
|
|
|
<div id="main">
|
|
|
|
<h1 class="page-title">Source: openpgp.js</h1>
|
|
|
|
|
|
|
|
|
|
|
|
<section>
|
|
<article>
|
|
<pre class="prettyprint source"><code>// GPG4Browsers - An OpenPGP implementation in javascript
|
|
// Copyright (C) 2011 Recurity Labs GmbH
|
|
//
|
|
// This library is free software; you can redistribute it and/or
|
|
// modify it under the terms of the GNU Lesser General Public
|
|
// License as published by the Free Software Foundation; either
|
|
// version 2.1 of the License, or (at your option) any later version.
|
|
//
|
|
// This library is distributed in the hope that it will be useful,
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// Lesser General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU Lesser General Public
|
|
// License along with this library; if not, write to the Free Software
|
|
// Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
|
|
|
|
/**
|
|
* @fileoverview The openpgp base module should provide all of the functionality
|
|
* to consume the openpgp.js library. All additional classes are documented
|
|
* for extending and developing on top of the base library.
|
|
*/
|
|
|
|
/**
|
|
* @requires cleartext
|
|
* @requires config
|
|
* @requires encoding/armor
|
|
* @requires enums
|
|
* @requires message
|
|
* @requires packet
|
|
* @module openpgp
|
|
*/
|
|
|
|
var armor = require('./encoding/armor.js'),
|
|
packet = require('./packet'),
|
|
enums = require('./enums.js'),
|
|
config = require('./config'),
|
|
message = require('./message.js'),
|
|
cleartext = require('./cleartext.js'),
|
|
key = require('./key.js');
|
|
|
|
|
|
/**
|
|
* Encrypts message text with keys
|
|
* @param {Array<module:key~Key>} keys array of keys, used to encrypt the message
|
|
* @param {String} text message as native JavaScript string
|
|
* @return {String} encrypted ASCII armored message
|
|
* @static
|
|
*/
|
|
function encryptMessage(keys, text) {
|
|
var msg = message.fromText(text);
|
|
msg = msg.encrypt(keys);
|
|
var armored = armor.encode(enums.armor.message, msg.packets.write());
|
|
return armored;
|
|
}
|
|
|
|
/**
|
|
* Signs message text and encrypts it
|
|
* @param {Array<module:key~Key>} publicKeys array of keys, used to encrypt the message
|
|
* @param {module:key~Key} privateKey private key with decrypted secret key data for signing
|
|
* @param {String} text message as native JavaScript string
|
|
* @return {String} encrypted ASCII armored message
|
|
* @static
|
|
*/
|
|
function signAndEncryptMessage(publicKeys, privateKey, text) {
|
|
var msg = message.fromText(text);
|
|
msg = msg.sign([privateKey]);
|
|
msg = msg.encrypt(publicKeys);
|
|
var armored = armor.encode(enums.armor.message, msg.packets.write());
|
|
return armored;
|
|
}
|
|
|
|
/**
|
|
* Decrypts message
|
|
* @param {module:key~Key} privateKey private key with decrypted secret key data
|
|
* @param {module:message~Message} message the message object with the encrypted data
|
|
* @return {(String|null)} decrypted message as as native JavaScript string
|
|
* or null if no literal data found
|
|
* @static
|
|
*/
|
|
function decryptMessage(privateKey, message) {
|
|
message = message.decrypt(privateKey);
|
|
return message.getText();
|
|
}
|
|
|
|
/**
|
|
* Decrypts message and verifies signatures
|
|
* @param {module:key~Key} privateKey private key with decrypted secret key data
|
|
* @param {Array<module:key~Key>} publicKeys public keys to verify signatures
|
|
* @param {module:message~Message} message the message object with signed and encrypted data
|
|
* @return {{text: String, signatures: Array<{keyid: module:type/keyid, valid: Boolean}>}}
|
|
* decrypted message as as native JavaScript string
|
|
* with verified signatures or null if no literal data found
|
|
* @static
|
|
*/
|
|
function decryptAndVerifyMessage(privateKey, publicKeys, message) {
|
|
var result = {};
|
|
message = message.decrypt(privateKey);
|
|
result.text = message.getText();
|
|
if (result.text) {
|
|
result.signatures = message.verify(publicKeys);
|
|
return result;
|
|
}
|
|
return null;
|
|
}
|
|
|
|
/**
|
|
* Signs a cleartext message
|
|
* @param {Array<module:key~Key>} privateKeys private key with decrypted secret key data to sign cleartext
|
|
* @param {String} text cleartext
|
|
* @return {String} ASCII armored message
|
|
* @static
|
|
*/
|
|
function signClearMessage(privateKeys, text) {
|
|
var cleartextMessage = new cleartext.CleartextMessage(text);
|
|
cleartextMessage.sign(privateKeys);
|
|
return cleartextMessage.armor();
|
|
}
|
|
|
|
/**
|
|
* Verifies signatures of cleartext signed message
|
|
* @param {Array<module:key~Key>} publicKeys public keys to verify signatures
|
|
* @param {module:cleartext~CleartextMessage} message cleartext message object with signatures
|
|
* @return {{text: String, signatures: Array<{keyid: module:type/keyid, valid: Boolean}>}}
|
|
* cleartext with status of verified signatures
|
|
* @static
|
|
*/
|
|
function verifyClearSignedMessage(publicKeys, message) {
|
|
var result = {};
|
|
if (!(message instanceof cleartext.CleartextMessage)) {
|
|
throw new Error('Parameter [message] needs to be of type CleartextMessage.');
|
|
}
|
|
result.text = message.getText();
|
|
result.signatures = message.verify(publicKeys);
|
|
return result;
|
|
}
|
|
|
|
/**
|
|
* Generates a new OpenPGP key pair. Currently only supports RSA keys.
|
|
* Primary and subkey will be of same type.
|
|
* @param {module:enums.publicKey} keyType to indicate what type of key to make.
|
|
* RSA is 1. See {@link http://tools.ietf.org/html/rfc4880#section-9.1}
|
|
* @param {Integer} numBits number of bits for the key creation. (should be 1024+, generally)
|
|
* @param {String} userId assumes already in form of "User Name <username@email.com>"
|
|
* @param {String} passphrase The passphrase used to encrypt the resulting private key
|
|
* @return {Object} {key: Array<module:key~Key>, privateKeyArmored: Array<String>, publicKeyArmored: Array<String>}
|
|
* @static
|
|
*/
|
|
function generateKeyPair(keyType, numBits, userId, passphrase) {
|
|
var result = {};
|
|
var newKey = key.generate(keyType, numBits, userId, passphrase);
|
|
result.key = newKey;
|
|
result.privateKeyArmored = newKey.armor();
|
|
result.publicKeyArmored = newKey.toPublic().armor();
|
|
return result;
|
|
}
|
|
|
|
exports.encryptMessage = encryptMessage;
|
|
exports.signAndEncryptMessage = signAndEncryptMessage;
|
|
exports.decryptMessage = decryptMessage;
|
|
exports.decryptAndVerifyMessage = decryptAndVerifyMessage;
|
|
exports.signClearMessage = signClearMessage;
|
|
exports.verifyClearSignedMessage = verifyClearSignedMessage;
|
|
exports.generateKeyPair = generateKeyPair;
|
|
</code></pre>
|
|
</article>
|
|
</section>
|
|
|
|
|
|
|
|
|
|
</div>
|
|
|
|
<nav>
|
|
<h2><a href="index.html">Index</a></h2><h3>Modules</h3><ul><li><a href="module-cleartext.html">cleartext</a></li><li><a href="module-config.html">config</a></li><li><a href="config.html">config/config</a></li><li><a href="localStorage.html">config/localStorage</a></li><li><a href="module-crypto.html">crypto</a></li><li><a href="cfb.html">crypto/cfb</a></li><li><a href="cipher.html">crypto/cipher</a></li><li><a href="aes.html">crypto/cipher/aes</a></li><li><a href="blowfish.html">crypto/cipher/blowfish</a></li><li><a href="cast5.html">crypto/cipher/cast5</a></li><li><a href="des.html">crypto/cipher/des</a></li><li><a href="twofish.html">crypto/cipher/twofish</a></li><li><a href="crypto.html">crypto/crypto</a></li><li><a href="hash.html">crypto/hash</a></li><li><a href="md5.html">crypto/hash/md5</a></li><li><a href="ripe-md.html">crypto/hash/ripe-md</a></li><li><a href="sha.html">crypto/hash/sha</a></li><li><a href="pkcs1.html">crypto/pkcs1</a></li><li><a href="public_key.html">crypto/public_key</a></li><li><a href="dsa.html">crypto/public_key/dsa</a></li><li><a href="elgamal.html">crypto/public_key/elgamal</a></li><li><a href="jsbn.html">crypto/public_key/jsbn</a></li><li><a href="rsa.html">crypto/public_key/rsa</a></li><li><a href="random.html">crypto/random</a></li><li><a href="signature.html">crypto/signature</a></li><li><a href="armor.html">encoding/armor</a></li><li><a href="base64.html">encoding/base64</a></li><li><a href="module-enums.html">enums</a></li><li><a href="module-key.html">key</a></li><li><a href="module-keyring.html">keyring</a></li><li><a href="keyring.html">keyring/keyring</a></li><li><a href="localstore.html">keyring/localstore</a></li><li><a href="module-message.html">message</a></li><li><a href="module-openpgp.html">openpgp</a></li><li><a href="module-packet.html">packet</a></li><li><a href="compressed.html">packet/compressed</a></li><li><a href="literal.html">packet/literal</a></li><li><a href="marker.html">packet/marker</a></li><li><a href="one_pass_signature.html">packet/one_pass_signature</a></li><li><a href="packet.html">packet/packet</a></li><li><a href="packetlist.html">packet/packetlist</a></li><li><a href="public_key_.html">packet/public_key</a></li><li><a href="public_key_encrypted_session_key.html">packet/public_key_encrypted_session_key</a></li><li><a href="public_subkey.html">packet/public_subkey</a></li><li><a href="secret_key.html">packet/secret_key</a></li><li><a href="secret_subkey.html">packet/secret_subkey</a></li><li><a href="signature_.html">packet/signature</a></li><li><a href="sym_encrypted_integrity_protected.html">packet/sym_encrypted_integrity_protected</a></li><li><a href="sym_encrypted_session_key.html">packet/sym_encrypted_session_key</a></li><li><a href="symmetrically_encrypted.html">packet/symmetrically_encrypted</a></li><li><a href="trust.html">packet/trust</a></li><li><a href="user_attribute.html">packet/user_attribute</a></li><li><a href="userid.html">packet/userid</a></li><li><a href="keyid.html">type/keyid</a></li><li><a href="mpi.html">type/mpi</a></li><li><a href="s2k.html">type/s2k</a></li><li><a href="module-util.html">util</a></li></ul><h3>Classes</h3><ul><li><a href="JXG.Util.html">JXG.Util</a></li><li><a href="module-cleartext-CleartextMessage.html">cleartext~CleartextMessage</a></li><li><a href="localStorage-LocalStorage.html">config/localStorage~LocalStorage</a></li><li><a href="keyring-Keyring.html">keyring/keyring~Keyring</a></li><li><a href="module-key-Key.html">key~Key</a></li><li><a href="module-key-SubKey.html">key~SubKey</a></li><li><a href="module-key-User.html">key~User</a></li><li><a href="module-message-Message.html">message~Message</a></li><li><a href="compressed-Compressed.html">packet/compressed~Compressed</a></li><li><a href="literal-Literal.html">packet/literal~Literal</a></li><li><a href="marker-Marker.html">packet/marker~Marker</a></li><li><a href="one_pass_signature-OnePassSignature.html">packet/one_pass_signature~OnePassSignature</a></li><li><a href="packetlist-Packetlist.html">packet/packetlist~Packetlist</a></li><li><a href="public_key_encrypted_session_key-PublicKeyEncryptedSessionKey.html">packet/public_key_encrypted_session_key~PublicKeyEncryptedSessionKey</a></li><li><a href="public_key-PublicKey.html">packet/public_key~PublicKey</a></li><li><a href="public_subkey-PublicSubkey.html">packet/public_subkey~PublicSubkey</a></li><li><a href="secret_key-SecretKey.html">packet/secret_key~SecretKey</a></li><li><a href="secret_subkey-SecretSubkey.html">packet/secret_subkey~SecretSubkey</a></li><li><a href="signature-Signature.html">packet/signature~Signature</a></li><li><a href="sym_encrypted_integrity_protected-SymEncryptedIntegrityProtected.html">packet/sym_encrypted_integrity_protected~SymEncryptedIntegrityProtected</a></li><li><a href="sym_encrypted_session_key-SymEncryptedSessionKey.html">packet/sym_encrypted_session_key~SymEncryptedSessionKey</a></li><li><a href="symmetrically_encrypted-SymmetricallyEncrypted.html">packet/symmetrically_encrypted~SymmetricallyEncrypted</a></li><li><a href="trust-Trust.html">packet/trust~Trust</a></li><li><a href="user_attribute-UserAttribute.html">packet/user_attribute~UserAttribute</a></li><li><a href="userid-Userid.html">packet/userid~Userid</a></li><li><a href="keyid-Keyid.html">type/keyid~Keyid</a></li><li><a href="mpi-MPI.html">type/mpi~MPI</a></li><li><a href="s2k-S2K.html">type/s2k~S2K</a></li></ul>
|
|
</nav>
|
|
|
|
<br clear="both">
|
|
|
|
<footer>
|
|
Documentation generated by <a href="https://github.com/jsdoc3/jsdoc">JSDoc 3.2.2</a> on Thu Jan 09 2014 02:24:28 GMT-0800 (PST)
|
|
</footer>
|
|
|
|
<script> prettyPrint(); </script>
|
|
<script src="scripts/linenumber.js"> </script>
|
|
</body>
|
|
</html>
|