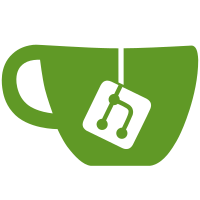
intersection.py - added tests (preparing for change vector->matfunc) clsolver2D.py - added stub for HH2S
168 lines
5.6 KiB
Plaintext
168 lines
5.6 KiB
Plaintext
TODO/WISH LIST
|
|
--------------
|
|
|
|
Code: Some bits of the code are ugly:
|
|
- vector module, matpy module and Numpy package, all offer similar
|
|
functionality. Use only one, get rid of two.
|
|
- more documentation!
|
|
|
|
Speed: The solver is currently much too slow. The problem is the pattern
|
|
matching algorithms that is used to find combinations of clusters that
|
|
can be rewritten/merged. Solutions:
|
|
- incremental pattern matching system (work in progress)
|
|
- compliled implementation (Psycho/C++/Haskell/???)
|
|
|
|
Rules: More rewrite rules to increase the problem domain:
|
|
- need 3d rule: merge of 2 ridids with 2 shared points (hinge) + hog with angle not on hinge points
|
|
- larger subproblems (octahedron, variable radius spheres/cylinders)
|
|
- new clusters types (N degrees of freedom)
|
|
|
|
Extentions:
|
|
- implement geometry: lines, planes, spheres, cylinders, tori (mappings to
|
|
cluster)
|
|
- constraints on parameters (equality, algebraic constraints)
|
|
- different variable domains (integers, reals, n-dimensional points,
|
|
surfaces, volumes, logic variables, lists)
|
|
|
|
Easy of use:
|
|
- move use_prototype flag to GeometricProblem (instead of in
|
|
GeometricSolver)
|
|
- check dimension of prototype points when adding to problem
|
|
|
|
|
|
BUGS:
|
|
|
|
- solver sometimes does not terminate - keeps adding merges where the result
|
|
cluster and one of the original clusters merge again and again. Problem
|
|
likely that a source cluster should be removed (reducdant) but isn't.
|
|
|
|
- following should be well-constraint, gives underconstrained (need extra rule/pattern)
|
|
def diamond_3d():
|
|
"""creates a diamond shape with point 'v1'...'v4' in 3D with one solution"""
|
|
L=10.0
|
|
problem = GeometricProblem(dimension=3)
|
|
problem.add_point('v1', vector([0.0, 0.0, 0.0]))
|
|
problem.add_point('v2', vector([-5.0, 5.0, 0.0]))
|
|
problem.add_point('v3', vector([5.0, 5.0, 0.0]))
|
|
problem.add_point('v4', vector([0.0, 10.0, 0.0]))
|
|
problem.add_constraint(DistanceConstraint('v1', 'v2', L))
|
|
problem.add_constraint(DistanceConstraint('v1', 'v3', L))
|
|
problem.add_constraint(DistanceConstraint('v2', 'v3', L))
|
|
problem.add_constraint(DistanceConstraint('v2', 'v4', L))
|
|
problem.add_constraint(DistanceConstraint('v3', 'v4', L))
|
|
# this bit of code constrains the points v1...v4 in a plane with point p above v1
|
|
problem.add_point('p', vector([0.0, 0.0, 1.0]))
|
|
problem.add_constraint(DistanceConstraint('v1', 'p', 1.0))
|
|
problem.add_constraint(AngleConstraint('v2','v1','p', math.pi/2))
|
|
problem.add_constraint(AngleConstraint('v3','v1','p', math.pi/2))
|
|
problem.add_constraint(AngleConstraint('v4','v1','p', math.pi/2))
|
|
|
|
- when fixed (by swapping v1 and v3 in last bit of code)
|
|
sometimes raises:
|
|
StandardError: more than one candidate prototype cluster for variable v2
|
|
or:
|
|
FixConstraint(v2=[-5.0, 5.0, 0.0]) not satisfied
|
|
FixConstraint(v1=[0.0, 0.0, 0.0]) not satisfied
|
|
|
|
- when solvin DAD type clusters, sometimes raises:
|
|
StandardError: cluster rigid#157388428(['v1', 'v2', 'v3']) already in clsolver
|
|
|
|
Debug output:
|
|
|
|
Solving....
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: a = v3
|
|
triplet2dad: c = v1
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: a = v3
|
|
triplet2dad: c = v1
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: start
|
|
triplet2dad: b = v2
|
|
triplet2dad: b in rigids
|
|
Traceback (most recent call last):
|
|
File "test.py", line 971, in <module>
|
|
t2d()
|
|
File "test.py", line 968, in test2d
|
|
test(dad_problem())
|
|
File "test.py", line 780, in test
|
|
solver = GeometricSolver(problem, use_prototype)
|
|
File "/home/rick/lib/python/geosolver/geometric.py", line 294, in __init__
|
|
self._add_constraint(con)
|
|
File "/home/rick/lib/python/geosolver/geometric.py", line 486, in _add_constraint
|
|
self.dr.add(hog)
|
|
File "/home/rick/lib/python/geosolver/clsolver.py", line 79, in add
|
|
self._process_new()
|
|
File "/home/rick/lib/python/geosolver/clsolver.py", line 363, in _process_new
|
|
self._add_method_complete(method)
|
|
File "/home/rick/lib/python/geosolver/clsolver.py", line 487, in _add_method_complete
|
|
self._add_cluster(output)
|
|
File "/home/rick/lib/python/geosolver/clsolver.py", line 236, in _add_cluster
|
|
raise StandardError, "cluster %s already in clsolver"%(str(newcluster))
|
|
StandardError: cluster rigid#157388428(['v1', 'v2', 'v3']) already in clsolver
|