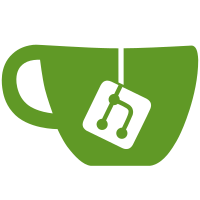
Support GUI scaling in much the same way as on Windows, where the OS setting ("org.gnome.desktop.interface.scaling-factor" times "...text-scaling-factor") determines the scale that is used for both graphics and GUI sizing. As I understand it, a complete solution requires porting to Gtk3. With Gtk2, the graphical part of a widget doesn't scale. Text and image labels should scale correctly, though.
60 lines
1.5 KiB
Racket
60 lines
1.5 KiB
Racket
#lang racket/base
|
|
(require ffi/unsafe
|
|
racket/class
|
|
"../../syntax.rkt"
|
|
"widget.rkt"
|
|
"window.rkt"
|
|
"utils.rkt"
|
|
"const.rkt"
|
|
"types.rkt")
|
|
|
|
(provide
|
|
(protect-out client-size-mixin))
|
|
|
|
;; ----------------------------------------
|
|
|
|
(define-signal-handler connect-size-allocate "size-allocate"
|
|
(_fun _GtkWidget _GtkAllocation-pointer -> _gboolean)
|
|
(lambda (gtk a)
|
|
(let ([wx (gtk->wx gtk)])
|
|
(when wx
|
|
(send wx save-client-size
|
|
(->normal (GtkAllocation-x a))
|
|
(->normal (GtkAllocation-y a))
|
|
(->normal (GtkAllocation-width a))
|
|
(->normal (GtkAllocation-height a)))))
|
|
#t))
|
|
|
|
(define (client-size-mixin %)
|
|
(class %
|
|
(init client-gtk)
|
|
|
|
(connect-size-allocate client-gtk)
|
|
|
|
(define client-x 0)
|
|
(define client-y 0)
|
|
|
|
(define/public (on-client-size w h) (void))
|
|
|
|
(define client-size-key #f)
|
|
|
|
(define/public (save-client-size x y w h)
|
|
;; Called in the Gtk event-loop thread
|
|
(set! client-x x)
|
|
(set! client-y y)
|
|
(when client-size-key (set-box! client-size-key #f))
|
|
(let ([key (box #t)])
|
|
(set! client-size-key key)
|
|
(queue-window-event this (lambda ()
|
|
(when (unbox key)
|
|
(internal-on-client-size w h)
|
|
(on-client-size w h))))))
|
|
|
|
(define/public (internal-on-client-size w h)
|
|
(void))
|
|
|
|
(define/override (get-client-delta)
|
|
(values client-x client-y))
|
|
|
|
(super-new)))
|