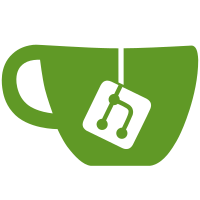
Fixed bigfloat functions that assumed (fixnum? x) means x fits in a _long (not true on Win64) Hopefully fixed dangling pointer errors that broke `math/bigfloat' on Win64. It apparently had no _long/_int mismatches, but GC on Win64 will run between creating an `_mpz' and using its value after passing it as an output argument to MPFR functions. That doesn't seem to happen on 64-bit Linux or Mac. No idea why, but Win64 exposed the problem so... that's good, I guess. Rewrote `rational->bigfloat' to not use GMP's rationals More/better bigfloat tests Added bigfloat stress test w/ weak leak detection Reenabled custodian shutdown callback that clears MPFR constants, because it seems to work now Removed `mpfr-available?' because it would only return non-#f
36 lines
993 B
Racket
36 lines
993 B
Racket
#lang racket
|
|
|
|
(require math/bigfloat
|
|
math/flonum
|
|
math/special-functions
|
|
math/private/bigfloat/bigfloat-hurwitz-zeta)
|
|
|
|
(collect-garbage)
|
|
(collect-garbage)
|
|
(collect-garbage)
|
|
(define start-mem (current-memory-use))
|
|
|
|
(define num 60)
|
|
|
|
(for ([x (in-range 1 num)])
|
|
(let ([x (* 0.25 x)])
|
|
(printf "x = ~v~n" x)
|
|
(for ([y (in-range 1 num)])
|
|
(let ([y (* 0.25 y)])
|
|
(define z (bigfloat->flonum (bfhurwitz-zeta (bf x) (bf y))))
|
|
(define err (flulp-error (flhurwitz-zeta x y) z))
|
|
(unless (err . <= . 5.0)
|
|
(printf "hurwitz-zeta ~v ~v; error = ~v ulps~n" x y err))))))
|
|
|
|
(collect-garbage)
|
|
(collect-garbage)
|
|
(collect-garbage)
|
|
(define end-mem (current-memory-use))
|
|
|
|
(define diff-mem (- end-mem start-mem))
|
|
(when (diff-mem . > . (* 2 1024 1024))
|
|
(printf "Significant higher memory use (more than 2MB):~n")
|
|
(printf " start-mem = ~v~n" start-mem)
|
|
(printf " end-mem = ~v~n" end-mem)
|
|
(printf " difference = ~v~n" diff-mem))
|