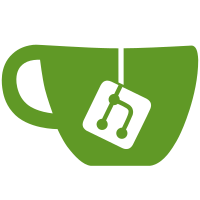
The eopl language is now racket-based rather than mzscheme-based. This test-suite, which was originally distributed on the book's web-site has been re-written in the new language. Changes include dropping all drscheme-init.scm and top.scm files. Remaining files were renamed to use the .rkt extension and edited to use the #lang syntax (instead of modulue). Require and provide forms were changed to reflect racket's syntax instead of mzscheme's (eg, only-in vs. only). Several occurrences of one-armed ifs were changed to use when and unless. All tests have been run successfully.
53 lines
1.3 KiB
Racket
Executable File
53 lines
1.3 KiB
Racket
Executable File
#lang eopl
|
|
|
|
;; builds environment interface, using data structures defined in
|
|
;; data-structures.rkt.
|
|
|
|
(require "data-structures.rkt")
|
|
|
|
(provide init-env empty-env extend-env apply-env)
|
|
|
|
;;;;;;;;;;;;;;;; initial environment ;;;;;;;;;;;;;;;;
|
|
|
|
;; init-env : () -> Env
|
|
;; usage: (init-env) = [i=1, v=5, x=10]
|
|
;; (init-env) builds an environment in which i is bound to the
|
|
;; expressed value 1, v is bound to the expressed value 5, and x is
|
|
;; bound to the expressed value 10.
|
|
;; Page: 69
|
|
|
|
(define init-env
|
|
(lambda ()
|
|
(extend-env
|
|
'i (num-val 1)
|
|
(extend-env
|
|
'v (num-val 5)
|
|
(extend-env
|
|
'x (num-val 10)
|
|
(empty-env))))))
|
|
|
|
;;;;;;;;;;;;;;;; environment constructors and observers ;;;;;;;;;;;;;;;;
|
|
|
|
(define empty-env
|
|
(lambda ()
|
|
(empty-env-record)))
|
|
|
|
(define empty-env?
|
|
(lambda (x)
|
|
(empty-env-record? x)))
|
|
|
|
(define extend-env
|
|
(lambda (sym val old-env)
|
|
(extended-env-record sym val old-env)))
|
|
|
|
(define apply-env
|
|
(lambda (env search-sym)
|
|
(if (empty-env? env)
|
|
(eopl:error 'apply-env "No binding for ~s" search-sym)
|
|
(let ((sym (extended-env-record->sym env))
|
|
(val (extended-env-record->val env))
|
|
(old-env (extended-env-record->old-env env)))
|
|
(if (eqv? search-sym sym)
|
|
val
|
|
(apply-env old-env search-sym))))))
|