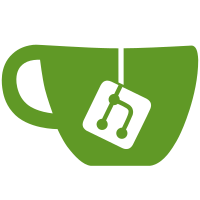
The eopl language is now racket-based rather than mzscheme-based. This test-suite, which was originally distributed on the book's web-site has been re-written in the new language. Changes include dropping all drscheme-init.scm and top.scm files. Remaining files were renamed to use the .rkt extension and edited to use the #lang syntax (instead of modulue). Require and provide forms were changed to reflect racket's syntax instead of mzscheme's (eg, only-in vs. only). Several occurrences of one-armed ifs were changed to use when and unless. All tests have been run successfully.
78 lines
1.9 KiB
Racket
Executable File
78 lines
1.9 KiB
Racket
Executable File
#lang eopl
|
|
|
|
(require "queues.rkt")
|
|
(require "data-structures.rkt") ; for continuation?
|
|
(require "lang.rkt") ; for expval?
|
|
|
|
(provide
|
|
initialize-scheduler!
|
|
set-final-answer!
|
|
|
|
time-expired?
|
|
decrement-timer!
|
|
|
|
place-on-ready-queue!
|
|
run-next-thread
|
|
|
|
)
|
|
|
|
;;;;;;;;;;;;;;;; the state ;;;;;;;;;;;;;;;;
|
|
|
|
;; components of the scheduler state:
|
|
|
|
(define the-ready-queue 'uninitialized)
|
|
(define the-final-answer 'uninitialized)
|
|
|
|
(define the-max-time-slice 'uninitialized)
|
|
(define the-time-remaining 'uninitialized)
|
|
|
|
;; initialize-scheduler! : Int -> Unspecified
|
|
(define initialize-scheduler!
|
|
(lambda (ticks)
|
|
(set! the-ready-queue (empty-queue))
|
|
(set! the-final-answer 'uninitialized)
|
|
(set! the-max-time-slice ticks)
|
|
(set! the-time-remaining the-max-time-slice)
|
|
))
|
|
|
|
;;;;;;;;;;;;;;;; the final answer ;;;;;;;;;;;;;;;;
|
|
|
|
;; place-on-ready-queue! : Thread -> Unspecified
|
|
;; Page: 184
|
|
(define place-on-ready-queue!
|
|
(lambda (th)
|
|
(set! the-ready-queue
|
|
(enqueue the-ready-queue th))))
|
|
|
|
;; run-next-thread : () -> FinalAnswer
|
|
;; Page: 184
|
|
(define run-next-thread
|
|
(lambda ()
|
|
(if (empty? the-ready-queue)
|
|
the-final-answer
|
|
(dequeue the-ready-queue
|
|
(lambda (first-ready-thread other-ready-threads)
|
|
(set! the-ready-queue other-ready-threads)
|
|
(set! the-time-remaining the-max-time-slice)
|
|
(first-ready-thread)
|
|
)))))
|
|
|
|
;; set-final-answer! : ExpVal -> Unspecified
|
|
;; Page: 184
|
|
(define set-final-answer!
|
|
(lambda (val)
|
|
(set! the-final-answer val)))
|
|
|
|
;; time-expired? : () -> Bool
|
|
;; Page: 184
|
|
(define time-expired?
|
|
(lambda ()
|
|
(zero? the-time-remaining)))
|
|
|
|
;; decrement-timer! : () -> Unspecified
|
|
;; Page: 184
|
|
(define decrement-timer!
|
|
(lambda ()
|
|
(set! the-time-remaining (- the-time-remaining 1))))
|
|
|