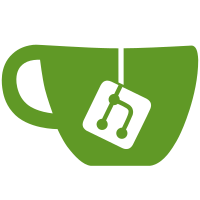
* Gram-Schmidt using vector type * QR decomposition * Operator 1-norm and maximum norm; stub for 2-norm and angle between subspaces (`matrix-basis-angle') * `matrix-absolute-error' and `matrix-relative-error'; also predicates based on them, such as `matrix-identity?' * Lots of shuffling code about * Types that can have contracts, and an exhaustive test to make sure every value exported by `math/matrix' has a contract when used in untyped code * Some more tests (still needs some)
21 lines
821 B
Racket
21 lines
821 B
Racket
#lang typed/racket/base
|
|
|
|
(require "matrix-types.rkt"
|
|
"matrix-constructors.rkt"
|
|
"matrix-arithmetic.rkt")
|
|
|
|
(provide matrix-expt)
|
|
|
|
(: matrix-expt : (Matrix Number) Integer -> (Matrix Number))
|
|
(define (matrix-expt a n)
|
|
(cond [(not (square-matrix? a)) (raise-argument-error 'matrix-expt "square-matrix?" 0 a n)]
|
|
[(negative? n) (raise-argument-error 'matrix-expt "Natural" 1 a n)]
|
|
[(zero? n) (identity-matrix (square-matrix-size a))]
|
|
[else
|
|
(let: loop : (Matrix Number) ([n : Positive-Integer n])
|
|
(cond [(= n 1) a]
|
|
[(= n 2) (matrix* a a)]
|
|
[(even? n) (let ([a^n/2 (matrix-expt a (quotient n 2))])
|
|
(matrix* a^n/2 a^n/2))]
|
|
[else (matrix* a (matrix-expt a (sub1 n)))]))]))
|