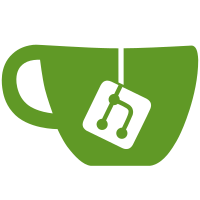
Fixed bigfloat functions that assumed (fixnum? x) means x fits in a _long (not true on Win64) Hopefully fixed dangling pointer errors that broke `math/bigfloat' on Win64. It apparently had no _long/_int mismatches, but GC on Win64 will run between creating an `_mpz' and using its value after passing it as an output argument to MPFR functions. That doesn't seem to happen on 64-bit Linux or Mac. No idea why, but Win64 exposed the problem so... that's good, I guess. Rewrote `rational->bigfloat' to not use GMP's rationals More/better bigfloat tests Added bigfloat stress test w/ weak leak detection Reenabled custodian shutdown callback that clears MPFR constants, because it seems to work now Removed `mpfr-available?' because it would only return non-#f
36 lines
1.3 KiB
Racket
36 lines
1.3 KiB
Racket
#lang racket/base
|
|
|
|
(require (for-syntax racket/base racket/syntax syntax/strip-context)
|
|
typed/racket/base
|
|
(only-in ffi/unsafe
|
|
ctype-sizeof
|
|
_long
|
|
_ulong))
|
|
|
|
(provide (all-defined-out))
|
|
|
|
(define (unsigned-max type) (- (expt 2 (* 8 (ctype-sizeof type))) 1))
|
|
(define (signed-min type) (- (expt 2 (- (* 8 (ctype-sizeof type)) 1))))
|
|
(define (signed-max type) (- (expt 2 (- (* 8 (ctype-sizeof type)) 1)) 1))
|
|
|
|
(define _long-min (signed-min _long))
|
|
(define _long-max (signed-max _long))
|
|
(define _ulong-max (unsigned-max _ulong))
|
|
|
|
(define (_ulong? n) (and (exact-integer? n) (<= 0 n _ulong-max)))
|
|
(define (_long? n) (and (exact-integer? n) (<= _long-min n _long-max)))
|
|
|
|
(define-syntax (req/prov-uniform-collection stx)
|
|
(syntax-case stx ()
|
|
[(_ module collection type)
|
|
(with-syntax ([require-it-name (datum->syntax stx (gensym 'require-it))])
|
|
(syntax/loc stx
|
|
(begin
|
|
(define-syntax (require-it-name stx1)
|
|
(syntax-case stx1 ()
|
|
[(require-it-name)
|
|
(with-syntax ([(obj (... ...)) (replace-context #'require-it-name collection)])
|
|
#'(begin (require/typed module [obj type] (... ...))
|
|
(provide obj (... ...))))]))
|
|
(require-it-name))))]))
|