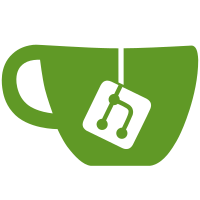
- `!!' now scans the same kind of data that `make-reader-graph' handles (except that hash-tables are not implemented) - this means no structs, no mpairs, and a bunch of other stuff - `!!!' is gone (lazy procedures are not wrapped) - dealing with multiple values moved into lazy/lazy.ss itself (and in the future everything will move in there) * Removed lazy/promise, and use scheme/promise instead. Also remove the docs for lazy/promise that were bogus (since scheme/promise *is* doing the same thing now). * Other adjustments to the docs. They should be considered incomplete now, and will need a major rewrite when the whole thing works again (multiple values things are just commented out for now). * Added a test macro and a quick test suite for lazy/promise. * The lazy tests are added to the nightly build tests svn: r11042
50 lines
1.5 KiB
Scheme
50 lines
1.5 KiB
Scheme
#lang scheme/base
|
|
|
|
(require "testing.ss" lazy/force)
|
|
|
|
;; lazy/force behavior
|
|
(test
|
|
(! 1) => 1
|
|
(! (! 1)) => 1
|
|
(! (~ 1)) => 1
|
|
(! (~ (~ (~ 1)))) => 1)
|
|
|
|
;; !list
|
|
(test
|
|
(!list (list 1 2 3)) => '(1 2 3)
|
|
(!list (~ (list 1 2 3))) => '(1 2 3)
|
|
(!list (~ (cons 1 (~ (cons 2 (~ (cons 3 (~ null)))))))) => '(1 2 3)
|
|
(!list 1) => 1 ; works on dotted lists
|
|
(!list (cons 1 2)) => '(1 . 2))
|
|
|
|
;; !!list
|
|
(test
|
|
(!!list (list 1 2 3)) => '(1 2 3)
|
|
(!!list (list (~ 1) (~ 2) (~ 3))) => '(1 2 3)
|
|
(!!list (list* (~ 1) (~ 2) (~ 3))) => '(1 2 . 3)
|
|
(!!list (~ (cons (~ 1) (~ (cons (~ 2) (~ (cons (~ 3) (~ null)))))))) => '(1 2 3)
|
|
(!!list (~ (cons (~ 1) (~ (list 2 3))))) => '(1 2 3)
|
|
(!!list (~ (cons (~ 1) (~ (list 2 (~ 3)))))) => '(1 2 3))
|
|
|
|
;; !!
|
|
(parameterize ([print-graph #t])
|
|
(test
|
|
(!! (~ (cons (~ 1) (~ (cons (~ 2) (~ (cons (~ 3) (~ null)))))))) => '(1 2 3)
|
|
(format "~s" (!! (letrec ([ones (~ (cons 1 (~ ones)))]) ones)))
|
|
=> "#0=(1 . #0#)"
|
|
(format "~s" (!! (letrec ([ones (~ (cons 1 (~ ones)))]) (list ones ones))))
|
|
=> "(#0=(1 . #0#) #0#)"
|
|
(format "~s" (!! (letrec ([x (vector 1 (~ x))]) x)))
|
|
=> "#0=#(1 #0#)"
|
|
(format "~s" (!! (letrec ([x (vector-immutable 1 (~ x))]) x)))
|
|
=> "#0=#(1 #0#)"
|
|
(format "~s" (!! (letrec ([x (box (~ x))]) x)))
|
|
=> "#0=#�#"
|
|
(format "~s" (!! (letrec ([x (box-immutable (~ x))]) x)))
|
|
=> "#0=#�#"
|
|
(format "~s" (!! (letrec ([x (make-prefab-struct 'foo 1 (~ x))]) x)))
|
|
=> "#0=#s(foo 1 #0#)"
|
|
))
|
|
|
|
(printf "All tests passed.\n")
|