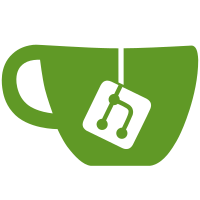
That is, when right-clicking on an imported identifier, if the file that has that identifier's definition is open and online check syntax has completed, then offer a "jump to definition" menu item that just jumps there with the already computed informtion. If the file isn't open or online check syntax hasn't completed, instead offer just to go to the file, without jumping to the definition also - things should generally work slightly better with submodules - jumping to identifiers should do a better job with scrolling, specifically it should scroll so the jumped-to identifier is about 20% from the top of the window (unless it was already visible, in which case no scrolling should occur)
67 lines
2.6 KiB
Racket
67 lines
2.6 KiB
Racket
#lang racket/base
|
|
(require racket/class
|
|
"local-member-names.rkt")
|
|
|
|
(define syncheck-annotations<%>
|
|
(interface ()
|
|
syncheck:find-source-object
|
|
syncheck:add-background-color
|
|
syncheck:add-require-open-menu
|
|
syncheck:add-docs-menu
|
|
syncheck:add-id-set
|
|
syncheck:add-arrow
|
|
syncheck:add-tail-arrow
|
|
syncheck:add-mouse-over-status
|
|
syncheck:add-jump-to-definition
|
|
syncheck:add-definition-target
|
|
syncheck:color-range
|
|
|
|
syncheck:add-rename-menu))
|
|
|
|
(define syncheck-text<%>
|
|
(interface (syncheck-annotations<%>)
|
|
syncheck:init-arrows
|
|
syncheck:clear-arrows
|
|
syncheck:arrows-visible?
|
|
syncheck:get-bindings-table
|
|
syncheck:jump-to-next-bound-occurrence
|
|
syncheck:jump-to-binding-occurrence
|
|
syncheck:jump-to-definition))
|
|
|
|
;; use this to communicate the frame being
|
|
;; syntax checked w/out having to add new
|
|
;; parameters to all of the functions
|
|
(define current-annotations (make-parameter #f))
|
|
|
|
(define annotations-mixin
|
|
(mixin () (syncheck-annotations<%>)
|
|
(define/public (syncheck:find-source-object stx) #f)
|
|
(define/public (syncheck:add-background-color source start end color) (void))
|
|
(define/public (syncheck:add-require-open-menu source start end key) (void))
|
|
(define/public (syncheck:add-id-set all-ids new-name-intereferes?)
|
|
(define fst (car all-ids))
|
|
(define src (list-ref fst 0))
|
|
(define sym
|
|
(cond
|
|
[(syntax? src) (syntax-e src)]
|
|
[(object? src) (send src get-text (list-ref fst 1) (list-ref fst 2))]
|
|
[else 'just-a-random-guess-as-to-a-good-id]))
|
|
(syncheck:add-rename-menu sym all-ids new-name-intereferes?))
|
|
(define/public (syncheck:add-rename-menu sym all-ids new-name-intereferes?) (void))
|
|
(define/public (syncheck:add-docs-menu text start-pos end-pos key the-label path definition-tag tag) (void))
|
|
(define/public (syncheck:add-arrow start-text start-pos-left start-pos-right
|
|
end-text end-pos-left end-pos-right
|
|
actual? level)
|
|
(void))
|
|
(define/public (syncheck:add-tail-arrow from-text from-pos to-text to-pos) (void))
|
|
(define/public (syncheck:add-mouse-over-status text pos-left pos-right str) (void))
|
|
(define/public (syncheck:add-jump-to-definition text start end id filename submods) (void))
|
|
(define/public (syncheck:add-definition-target source pos-left pos-right id mods) (void))
|
|
(define/public (syncheck:color-range source start finish style-name) (void))
|
|
(super-new)))
|
|
|
|
(provide syncheck-text<%>
|
|
syncheck-annotations<%>
|
|
current-annotations
|
|
annotations-mixin)
|