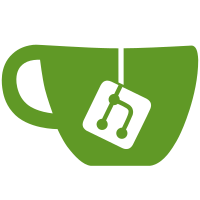
I started from tabs that are not on the beginning of lines, and in several places I did further cleanings. If you're worried about knowing who wrote some code, for example, if you get to this commit in "git blame", then note that you can use the "-w" flag in many git commands to ignore whitespaces. For example, to see per-line authors, use "git blame -w <file>". Another example: to see the (*much* smaller) non-whitespace changes in this (or any other) commit, use "git log -p -w -1 <sha1>".
45 lines
1.4 KiB
Racket
45 lines
1.4 KiB
Racket
#lang racket/load
|
|
|
|
(module a racket/base
|
|
(require racket/contract
|
|
(only-in racket/list first second rest empty?)
|
|
(prefix-in old: (only-in racket/list argmax)))
|
|
|
|
(define (argmax f lov)
|
|
(define r (old:argmax f lov))
|
|
(if (and (pair? r) (eq? (cadr r) 'bananas))
|
|
'(3 oranges)
|
|
r)) ;; yet another bug
|
|
|
|
(provide
|
|
(contract-out
|
|
[argmax
|
|
(->i ([f (-> any/c real?)] [lov (and/c pair? list?)]) ()
|
|
(r (f lov)
|
|
(lambda (r)
|
|
(define f@r (f r))
|
|
(and (for/and ((v lov)) (>= f@r (f v)))
|
|
(eq? (first (memf (lambda (v) (= (f v) f@r)) lov))
|
|
r)))))])))
|
|
|
|
(module b racket/base
|
|
(require 'a)
|
|
(require racket/contract/private/blame)
|
|
;; --- copied from version 1 ---
|
|
(with-handlers ([exn:fail:contract:blame? void])
|
|
(printf "*** 0: ~s\n" (argmax car '())))
|
|
(with-handlers ([exn:fail:contract:blame? void])
|
|
(printf "*** 1: ~s\n" (argmax car '())))
|
|
(with-handlers ([exn:fail:contract:blame? void])
|
|
(printf "*** 2: ~s\n" (argmax car '((apples 3)))))
|
|
(printf "3: ~s\n" (argmax car '((3 apples) (3 oranges))))
|
|
;; --- copies from version 2 ---
|
|
(printf "4: ~s\n" (argmax sqrt '(1)))
|
|
(printf "5: ~s\n" (argmax car '((3 apples) (3 oranges))))
|
|
;; --- new tests ---
|
|
(with-handlers ([exn:fail:contract:blame? void])
|
|
(printf "***6: ~s\n" (argmax car '((3 bananas) (3 oranges))))))
|
|
|
|
|
|
(require 'b)
|