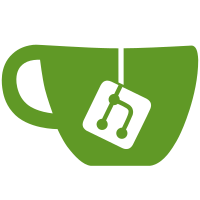
of a supertype and subtype, where the subtype was not yet added to the tenv. Had to hack to get around that one. Also little problems like the fact that list can be captured by the user program, so we can't use that -- used list* (with a null at the end) and null (for empty lists) instead. Since the power was down and I couldn't get the earlier stuff committed, I have even more changes. Bug-fixes, mostly, though now top-level functions that are defined consecutively are mutually recursive as they should be. svn: r300
25 lines
465 B
Plaintext
25 lines
465 B
Plaintext
type EvenOdd {
|
|
bool even(int);
|
|
bool odd(int);
|
|
}
|
|
|
|
// The following class tests mutually recursive methods.
|
|
|
|
class EvenOddC() : EvenOdd impl EvenOdd {
|
|
bool even(int n) {
|
|
cond {
|
|
n == 0 => return true;
|
|
n < 0 => return even(-n);
|
|
else return odd(n - 1);
|
|
};
|
|
}
|
|
bool odd(int n) {
|
|
cond {
|
|
n == 0 => return false;
|
|
n < 0 => return odd(-n);
|
|
else return even(n - 1);
|
|
};
|
|
}
|
|
export EvenOdd : even, odd;
|
|
}
|