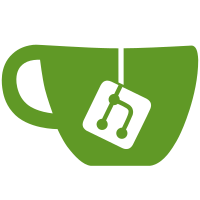
included in the compiled files. (also, misc minor cleanups notably a new exercise in tut.scrbl) closes PR 12547 --- there are still a few uses left, but they do not seem to be coming from Redex proper: - /Users/robby/git/plt/collects/racket/private/map.rkt still appears in a bunch of places (there is a separate PR for that I believe), and - /Users/robby/git/plt/collects/redex/../private/reduction-semantics.rkt appears in tl-test.rkt, but I do not see how it is coming in via Redex code, so hopefully one of the other PRs that Eli submitted is the real cause. If not, I'll revisit later
60 lines
1.3 KiB
Racket
60 lines
1.3 KiB
Racket
#lang racket
|
|
(require redex)
|
|
|
|
(define-language lang
|
|
(e (binop e e)
|
|
(sqrt e)
|
|
number)
|
|
(binop +
|
|
-
|
|
*
|
|
/)
|
|
|
|
(e-ctxt (binop e e-ctxt)
|
|
(binop e-ctxt e)
|
|
(sqrt e-ctxt)
|
|
hole)
|
|
(v number))
|
|
|
|
(define reductions
|
|
(reduction-relation
|
|
lang
|
|
(c--> (+ number_1 number_2)
|
|
,(+ (term number_1) (term number_2))
|
|
"add")
|
|
(c--> (- number_1 number_2)
|
|
,(- (term number_1) (term number_2))
|
|
"subtract")
|
|
(c--> (* number_1 number_2)
|
|
,(* (term number_1) (term number_2))
|
|
"multiply")
|
|
(c--> (/ number_1 number_2)
|
|
,(/ (term number_1) (term number_2))
|
|
"divide")
|
|
(c--> (sqrt number_1)
|
|
,(sqrt (term number_1))
|
|
"sqrt")
|
|
with
|
|
[(--> (in-hole e-ctxt_1 a) (in-hole e-ctxt_1 b))
|
|
(c--> a b)]))
|
|
|
|
(define traces-file
|
|
(make-temporary-file "traces~a.ps"))
|
|
|
|
(traces/ps reductions (term (- (* (sqrt 36) (/ 1 2)) (+ 1 2)))
|
|
traces-file)
|
|
|
|
;; Check for the command line flag --no-print
|
|
;; If it's set, don't print the temporary file name,
|
|
;; This flag is so that DrDr can avoid seeing a change here.
|
|
;; -- samth
|
|
(define print-name?
|
|
(let ([print? #t])
|
|
(command-line
|
|
#:once-each
|
|
["--no-print" "omit printing of file name" (set! print? #f)])
|
|
print?))
|
|
|
|
(when print-name?
|
|
(printf "Traces are in ~a\n" traces-file))
|