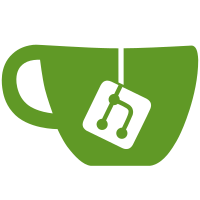
Clean up the use of styles for alignment and the generated instances of style names. In the process, remove some padding for HTML output, and make the rendering more configurable (especially for Latex, based on suggestions by Keven Tew). The way that nested flows are generated can be different than before, so these changes risk breaking some existing uses of `scriblib/figure'. The changes especially likely break uses of `scriblib/figure' with output configured through overiding .css/.tex definitions. But the old pile of styles/macros was inconsistent and broken in various ways, so hopefully the changes are an improvement overall. original commit: 8862a44f149e9a8fb975f63147730cb19a4a7931
107 lines
3.9 KiB
Racket
107 lines
3.9 KiB
Racket
#lang scheme/base
|
|
|
|
(require scribble/manual
|
|
scribble/core
|
|
scribble/decode
|
|
scribble/html-properties
|
|
scribble/latex-properties
|
|
setup/main-collects
|
|
"private/counter.rkt")
|
|
|
|
(provide figure
|
|
figure*
|
|
figure**
|
|
figure-here
|
|
Figure-target
|
|
Figure-ref
|
|
figure-ref
|
|
left-figure-style
|
|
center-figure-style
|
|
right-figure-style
|
|
(rename-out [left-figure-style left]))
|
|
|
|
(define figure-style-extras
|
|
(let ([abs (lambda (s)
|
|
(path->main-collects-relative
|
|
(collection-file-path s "scriblib")))])
|
|
(list (make-css-addition (abs "figure.css"))
|
|
(make-tex-addition (abs "figure.tex")))))
|
|
|
|
;; outer layer:
|
|
(define herefigure-style (make-style "Herefigure" figure-style-extras))
|
|
(define figure-style (make-style "Figure" figure-style-extras))
|
|
(define figuremulti-style (make-style "FigureMulti" figure-style-extras))
|
|
(define figuremultiwide-style (make-style "FigureMultiWide" figure-style-extras))
|
|
|
|
;; middle layer:
|
|
(define center-figure-style (make-style "Centerfigure" figure-style-extras))
|
|
(define left-figure-style (make-style "Leftfigure" figure-style-extras))
|
|
(define right-figure-style (make-style "Rightfigure" figure-style-extras))
|
|
|
|
;; inner layer:
|
|
(define figureinside-style (make-style "FigureInside" figure-style-extras))
|
|
|
|
(define legend-style (make-style "Legend" figure-style-extras))
|
|
|
|
(define centertext-style (make-style "Centertext" figure-style-extras))
|
|
|
|
(define (make-figure-ref c s)
|
|
(element (style "FigureRef" (list* (command-extras (list s))
|
|
figure-style-extras))
|
|
c))
|
|
(define (make-figure-target c s)
|
|
(element (style "FigureTarget" (cons (command-extras (list s))
|
|
figure-style-extras))
|
|
c))
|
|
|
|
(define (figure tag caption #:style [style center-figure-style] . content)
|
|
(figure-helper figure-style style tag caption content))
|
|
|
|
(define (figure-here tag caption #:style [style center-figure-style] . content)
|
|
(figure-helper herefigure-style style tag caption content))
|
|
|
|
(define (figure* tag caption #:style [style center-figure-style] . content)
|
|
(figure-helper figuremulti-style style tag caption content))
|
|
(define (figure** tag caption #:style [style center-figure-style] . content)
|
|
(figure-helper figuremultiwide-style style tag caption content))
|
|
|
|
(define (figure-helper figure-style content-style tag caption content)
|
|
(make-nested-flow
|
|
figure-style
|
|
(list
|
|
(make-nested-flow
|
|
content-style
|
|
(list (make-nested-flow figureinside-style (decode-flow content))))
|
|
(make-paragraph
|
|
centertext-style
|
|
(list (make-element legend-style (list (Figure-target tag) caption)))))))
|
|
|
|
(define figures (new-counter "figure"
|
|
#:target-wrap make-figure-target
|
|
#:ref-wrap make-figure-ref))
|
|
(define (Figure-target tag)
|
|
(counter-target figures tag "Figure" ": "))
|
|
|
|
(define (ref-proc initial)
|
|
(case-lambda
|
|
[(tag)
|
|
(make-element #f (list (counter-ref figures tag (string-append initial "igure"))))]
|
|
[(tag1 tag2)
|
|
(make-element #f (list (counter-ref figures tag1 (string-append initial "igures"))
|
|
" and "
|
|
(counter-ref figures tag2 #f)))]
|
|
[(tag . tags)
|
|
(make-element #f (cons (counter-ref figures tag (string-append initial "igures"))
|
|
(let loop ([tags tags])
|
|
(cond
|
|
[(null? (cdr tags))
|
|
(list ", and "
|
|
(counter-ref figures (car tags) #f))]
|
|
[else
|
|
(list* ", "
|
|
(counter-ref figures (car tags) #f)
|
|
(loop (cdr tags)))]))))]))
|
|
|
|
(define Figure-ref (ref-proc "F"))
|
|
(define figure-ref (ref-proc "f"))
|