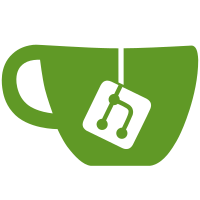
Summary: Implicit groups are objects that act like groups but don't have brackets around them. This is used for things like sizing functions or font-change functions that can occur in the middle of the group, but act like they apply to a group after them which stops when the current group stops. E.g. `Hello {world \Large hello} world` produces normal, normal, Large, normal text. (Note, I just came up with the name implicit group, I don't think this is actually how it is parsed in LaTeX but it fits nicely with the current parsing scheme and seems to work well). For now, apply this to the sizing functions (we don't have any other functions that act like this). Also note that this doesn't really do much practically because we limit sizing functions to only be on the top level of the expression, but it does let people do `\Large x` and get a large `x`, without having to add braces. Test Plan: - Run the tests, see they work - Make sure `abc \Large abc` looks correct Reviewers: alpert Reviewed By: alpert Differential Revision: http://phabricator.khanacademy.org/D10876
63 lines
1.6 KiB
JavaScript
63 lines
1.6 KiB
JavaScript
function Options(style, size, color, deep, parentStyle, parentSize) {
|
|
this.style = style;
|
|
this.color = color;
|
|
this.size = size;
|
|
|
|
// TODO(emily): Get rid of deep when we can actually use sizing everywhere
|
|
if (deep === undefined) {
|
|
deep = false;
|
|
}
|
|
this.deep = deep;
|
|
|
|
if (parentStyle === undefined) {
|
|
parentStyle = style;
|
|
}
|
|
this.parentStyle = parentStyle;
|
|
|
|
if (parentSize === undefined) {
|
|
parentSize = size;
|
|
}
|
|
this.parentSize = parentSize;
|
|
}
|
|
|
|
Options.prototype.withStyle = function(style) {
|
|
return new Options(style, this.size, this.color, this.deep,
|
|
this.style, this.size);
|
|
};
|
|
|
|
Options.prototype.withSize = function(size) {
|
|
return new Options(this.style, size, this.color, this.deep,
|
|
this.style, this.size);
|
|
};
|
|
|
|
Options.prototype.withColor = function(color) {
|
|
return new Options(this.style, this.size, color, this.deep,
|
|
this.style, this.size);
|
|
};
|
|
|
|
Options.prototype.deepen = function() {
|
|
return new Options(this.style, this.size, this.color, true,
|
|
this.parentStyle, this.parentSize);
|
|
};
|
|
|
|
Options.prototype.reset = function() {
|
|
return new Options(this.style, this.size, this.color, this.deep,
|
|
this.style, this.size);
|
|
};
|
|
|
|
var colorMap = {
|
|
"katex-blue": "#6495ed",
|
|
"katex-orange": "#ffa500",
|
|
"katex-pink": "#ff00af",
|
|
"katex-red": "#df0030",
|
|
"katex-green": "#28ae7b",
|
|
"katex-gray": "gray",
|
|
"katex-purple": "#9d38bd"
|
|
};
|
|
|
|
Options.prototype.getColor = function() {
|
|
return colorMap[this.color] || this.color;
|
|
};
|
|
|
|
module.exports = Options;
|