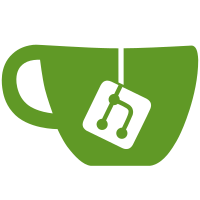
Summary: Right now, when the size gets bigger, this still doesn't work, so there's a check to prevent that. However, functions that go smaller (like `\small`, `\tiny`, etc) do work. Also, we can't seem to use the sizing functions inside of fractions (so something like `\dfrac{\small\frac{x}{y}}{z}` doesn't work). However, the most prominent use case is `\small` as the outer-most object, so this is still helpful. This commit has the parsing and stuff to handle all of it, but it'll throw an error if you try to do something that doesn't work. (For the record, "doesn't work" means "looks bad", not "throws an unexpected error"). Test Plan: Make sure things like `\small x` work, and things like `\Huge x` and `\frac{\small x}{y}` don't. Reviewers: alpert Reviewed By: alpert Differential Revision: http://phabricator.khanacademy.org/D3619
44 lines
1.1 KiB
JavaScript
44 lines
1.1 KiB
JavaScript
function Options(style, size, color, depth, parentStyle, parentSize) {
|
|
this.style = style;
|
|
this.color = color;
|
|
this.size = size;
|
|
|
|
// TODO(emily): Get rid of depth when we can actually use sizing everywhere
|
|
if (!depth) {
|
|
depth = 0;
|
|
}
|
|
this.depth = depth;
|
|
|
|
if (!parentStyle) {
|
|
parentStyle = style;
|
|
}
|
|
this.parentStyle = parentStyle;
|
|
|
|
if (!parentSize) {
|
|
parentSize = size;
|
|
}
|
|
this.parentSize = parentSize;
|
|
}
|
|
|
|
Options.prototype.withStyle = function(style) {
|
|
return new Options(style, this.size, this.color, this.depth + 1,
|
|
this.style, this.size);
|
|
};
|
|
|
|
Options.prototype.withSize = function(size) {
|
|
return new Options(this.style, size, this.color, this.depth + 1,
|
|
this.style, this.size);
|
|
};
|
|
|
|
Options.prototype.withColor = function(color) {
|
|
return new Options(this.style, this.size, color, this.depth + 1,
|
|
this.style, this.size);
|
|
};
|
|
|
|
Options.prototype.reset = function() {
|
|
return new Options(this.style, this.size, this.color, this.depth + 1,
|
|
this.style, this.size);
|
|
};
|
|
|
|
module.exports = Options;
|