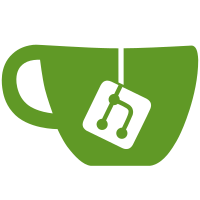
Make error messages from the lexing and parsing stages be a bit more helpful. If provided with the input and a position, the error will display the error position, and the nearby input with the error position underlined (yay combining marks). Also, standardize the errors a bit (remove doubled "Error:" strings) Test plan: - Make sure the errors look totally sweet (before: {F15602}, after: {F15603}) - Trigger every error (that can be triggered) in Parser, Lexer, and buildTree using the inputs: `a^` `a_` `a^x^x` `a_x_x` `\color f` `\blue ` `\Huge` `\llap` `\text` `\dfrac` `\dfrac{x}` `\d` `\blue{` `\color{#f` `{\Huge{x}}` - See that the tests still work Auditors: alpert
30 lines
942 B
JavaScript
30 lines
942 B
JavaScript
function ParseError(message, lexer, position) {
|
|
var error = "KaTeX parse error: " + message;
|
|
|
|
if (lexer !== undefined && position !== undefined) {
|
|
// If we have the input and a position, make the error a bit fancier
|
|
// Prepend some information
|
|
error += " at position " + position + ": ";
|
|
|
|
// Get the input
|
|
var input = lexer._input;
|
|
// Insert a combining underscore at the correct position
|
|
input = input.slice(0, position) + "\u0332" +
|
|
input.slice(position);
|
|
|
|
// Extract some context from the input and add it to the error
|
|
var begin = Math.max(0, position - 15);
|
|
var end = position + 15;
|
|
error += input.slice(begin, end);
|
|
}
|
|
|
|
var self = new Error(error);
|
|
self.name = "ParseError";
|
|
self.__proto__ = ParseError.prototype;
|
|
return self;
|
|
}
|
|
|
|
ParseError.prototype.__proto__ = Error.prototype;
|
|
|
|
module.exports = ParseError;
|