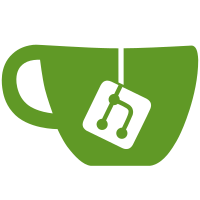
Summary: Upon switching over from ttf metrics to TeX metrics, we lost metrics for a couple of the characters that are dynamically generated by TeX. Thus TeX doesn't have metrics for them, but our fonts do have the characters because the MathJax scripts also dynamically build them. This adds the ability to extract metrics from the generated font files so that we can use the generated characters correctly. A better solution would be to dynamically generate the characters ourselves, but that is much harder, and will be left to a future time. Test Plan: - Make sure typing "\neq \cong \text{ }" produces no warnings in the console. - Make sure huxley screenshots look the same Reviewers: alpert Reviewed By: alpert Differential Revision: http://phabricator.khanacademy.org/D13107
48 lines
1.1 KiB
Python
Executable File
48 lines
1.1 KiB
Python
Executable File
#!/usr/bin/env python
|
|
|
|
import fontforge
|
|
import sys
|
|
import json
|
|
|
|
metrics_to_extract = {
|
|
"Main-Regular": [
|
|
u"\u2260",
|
|
u"\u2245",
|
|
u"\u0020",
|
|
u"\u00a0",
|
|
]
|
|
}
|
|
|
|
|
|
def main():
|
|
start_json = json.load(sys.stdin)
|
|
|
|
for font, chars in metrics_to_extract.iteritems():
|
|
fontInfo = fontforge.open("../static/fonts/KaTeX_" + font + ".ttf")
|
|
|
|
for glyph in fontInfo.glyphs():
|
|
try:
|
|
char = unichr(glyph.unicode)
|
|
except ValueError:
|
|
continue
|
|
|
|
if char in chars:
|
|
_, depth, _, height = glyph.boundingBox()
|
|
|
|
depth = -depth
|
|
|
|
# TODO(emily): Figure out a real way to calculate this
|
|
italic = 0
|
|
|
|
start_json[font][ord(char)] = {
|
|
height: height / fontInfo.em,
|
|
depth: depth / fontInfo.em,
|
|
italic: italic / fontInfo.em,
|
|
}
|
|
|
|
sys.stdout.write(
|
|
json.dumps(start_json, separators=(',', ':'), sort_keys=True))
|
|
|
|
if __name__ == "__main__":
|
|
main()
|