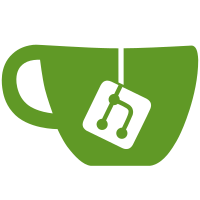
We don't need to allow auto updates for now. Such approach may need to be changed if pusher can deliver new jobs, but this should work for now.
54 lines
1.7 KiB
CoffeeScript
54 lines
1.7 KiB
CoffeeScript
require 'travis/model'
|
|
|
|
@Travis.Build = Travis.Model.extend
|
|
eventType: DS.attr('string')
|
|
repositoryId: DS.attr('number')
|
|
commitId: DS.attr('number')
|
|
|
|
state: DS.attr('string')
|
|
number: DS.attr('number')
|
|
branch: DS.attr('string')
|
|
message: DS.attr('string')
|
|
result: DS.attr('number')
|
|
duration: DS.attr('number')
|
|
startedAt: DS.attr('string')
|
|
finishedAt: DS.attr('string')
|
|
|
|
repository: DS.belongsTo('Travis.Repository')
|
|
commit: DS.belongsTo('Travis.Commit')
|
|
jobs: DS.hasMany('Travis.Job', key: 'job_ids')
|
|
|
|
config: (->
|
|
Travis.Helpers.compact(@get('data.config'))
|
|
).property('data.config')
|
|
|
|
isMatrix: (->
|
|
@get('data.job_ids.length') > 1
|
|
).property('data.job_ids.length')
|
|
|
|
requiredJobs: (->
|
|
@get('jobs').filter (data) -> !data.get('allow_failure')
|
|
).property('jobs.@each.allow_failure')
|
|
|
|
allowedFailureJobs: (->
|
|
@get('jobs').filter (data) -> data.get('allow_failure')
|
|
).property('jobs.@each.allow_failure')
|
|
|
|
configKeys: (->
|
|
return [] unless config = @get('config')
|
|
keys = $.intersect($.keys(config), Travis.CONFIG_KEYS)
|
|
headers = (I18n.t(key) for key in ['build.job', 'build.duration', 'build.finished_at'])
|
|
$.map(headers.concat(keys), (key) -> return $.camelize(key))
|
|
).property('config')
|
|
|
|
tick: ->
|
|
@notifyPropertyChange 'duration'
|
|
@notifyPropertyChange 'finished_at'
|
|
|
|
@Travis.Build.reopenClass
|
|
byRepositoryId: (id, parameters) ->
|
|
@find($.extend(parameters || {}, repository_id: id, orderBy: 'number DESC'))
|
|
|
|
olderThanNumber: (id, build_number) ->
|
|
@find(url: '/repositories/' + id + '/builds.json?bare=true&after_number=' + build_number, repository_id: id, orderBy: 'number DESC')
|