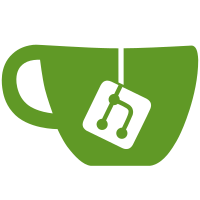
This commit fixes handling of branches when using both V3 and V2. The changes include: * proper definition of relationships that reflect V3 structure, so for example build belongs to a branch * setting up inverse records for some of the relationships. without doing that Ember Data can handle relationships in a surprising way, for example if the same record is referenced in 2 places in a belongsTo relationship, Ember Data will remove one of the references without proper inverse definitions * we need to add id when extracting branch as a relationship. Ember Data expects all of the relationships to have an id * lastly, we need to mimic the structure of the V3 API in V2 payloads, so for a build payload I'm now creating a branch record
86 lines
2.1 KiB
JavaScript
86 lines
2.1 KiB
JavaScript
import Ember from 'ember';
|
|
import V2FallbackSerializer from 'travis/serializers/v2_fallback';
|
|
|
|
var Serializer = V2FallbackSerializer.extend({
|
|
isNewSerializerAPI: true,
|
|
attrs: {
|
|
_config: {
|
|
key: 'config'
|
|
},
|
|
_finished_at: {
|
|
key: 'finished_at'
|
|
},
|
|
_started_at: {
|
|
key: 'started_at'
|
|
},
|
|
_duration: {
|
|
key: 'duration'
|
|
}
|
|
},
|
|
|
|
extractRelationships: function(modelClass, resourceHash) {
|
|
var result;
|
|
result = this._super(modelClass, resourceHash);
|
|
return result;
|
|
},
|
|
|
|
normalizeArrayResponse: function(store, primaryModelClass, payload, id, requestType) {
|
|
var result;
|
|
if (payload.commits) {
|
|
payload.builds.forEach(function(build) {
|
|
var commit, commit_id;
|
|
commit_id = build.commit_id;
|
|
if (commit = payload.commits.findBy('id', commit_id)) {
|
|
build.commit = commit;
|
|
return delete build.commit_id;
|
|
}
|
|
});
|
|
}
|
|
return this._super.apply(this, arguments);
|
|
},
|
|
|
|
keyForV2Relationship: function(key, typeClass, method) {
|
|
if(key === 'jobs') {
|
|
return 'job_ids';
|
|
} else if (key === 'repo') {
|
|
return 'repository_id';
|
|
} else if (key === 'commit') {
|
|
return key;
|
|
} else {
|
|
return this._super.apply(this, arguments);
|
|
}
|
|
},
|
|
|
|
normalize: function(modelClass, resourceHash) {
|
|
var data, href, id, repoId, result;
|
|
|
|
// TODO: remove this after switching to V3 entirely
|
|
if(!resourceHash['@type']) {
|
|
let build = resourceHash.build,
|
|
commit = resourceHash.commit;
|
|
let branch = {
|
|
name: commit.branch,
|
|
default_branch: build.is_on_default_branch,
|
|
"@href": `/repo/${build.repository_id}/branch/${commit.branch}`
|
|
};
|
|
resourceHash.build.branch = branch;
|
|
}
|
|
|
|
result = this._super(modelClass, resourceHash);
|
|
|
|
data = result.data;
|
|
|
|
if (repoId = resourceHash.repository_id) {
|
|
data.attributes.repositoryId = repoId;
|
|
} else if (resourceHash.repository) {
|
|
if (href = resourceHash.repository['@href']) {
|
|
id = href.match(/\d+/)[0];
|
|
data.attributes.repositoryId = id;
|
|
}
|
|
}
|
|
return result;
|
|
}
|
|
});
|
|
|
|
export default Serializer;
|