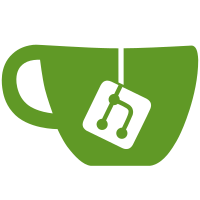
Prevent the static contract optimizer from changing constructors under `or/sc`. i.e., for static contracts of the form `(or/sc other-scs ...)`, the optimizer cannot optimize any of the `other-scs ...` to `any/sc` but it can optimize sub-contracts of the `other-scs ...` Example: `(or/sc set?/sc (box/sc set?/sc))` in a trusted position now optimizes to itself, instead of `any/sc` Optimization can resume under a sub-contract that represents a "heavy" type constructor. (I mean, `U` is a type constructor but it's not "heavy" like that.)
23 lines
399 B
Racket
23 lines
399 B
Racket
#lang typed/racket/base
|
|
|
|
(module u racket/base
|
|
(define (f b)
|
|
(set-box! b "hello"))
|
|
(provide f))
|
|
|
|
(define-type Maybe-Box (U #f (Boxof Integer)))
|
|
|
|
(require/typed 'u (f (-> Maybe-Box Void)))
|
|
|
|
(define b : Maybe-Box (box 4))
|
|
|
|
(module+ test
|
|
(require typed/rackunit)
|
|
|
|
(check-exn exn:fail:contract?
|
|
(λ () (f b)))
|
|
|
|
(check-equal?
|
|
(if (box? b) (+ 1 (unbox b)) (error 'deadcode))
|
|
5))
|